Developing Games using RingRayLib
In this chapter we will learn how to use the RingRayLib extension.
Introduction
RingRayLib is an extension for the RayLib game programming library.
Also RayGUI functions are supported by this extension.
Basic Window
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [core] example - basic window")
SetTargetFPS(60)
while !WindowShouldClose()
BeginDrawing()
ClearBackground(RED)
DrawText("Congrats! You created your first window!", 190, 200, 20, WHITE)
EndDrawing()
end
CloseWindow()
Screen Shot:
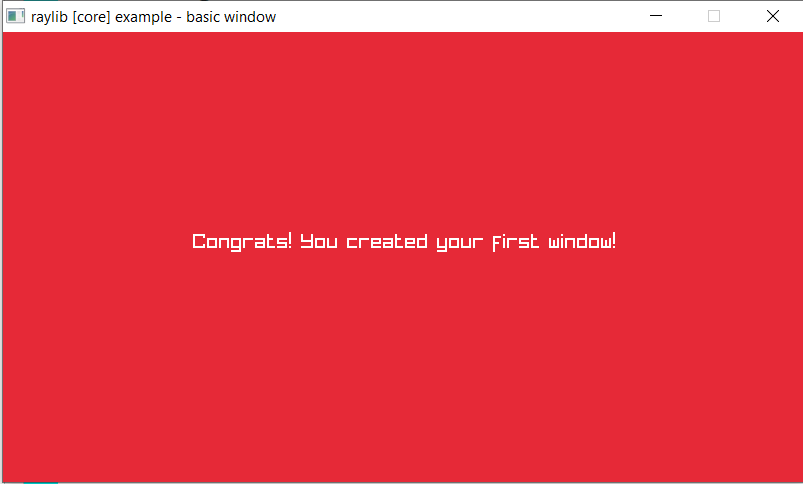
Input Keys
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [core] example - keyboard input")
ballPosition = Vector2(screenWidth/2, screenHeight/2)
SetTargetFPS(60)
while !WindowShouldClose()
if IsKeyDown(KEY_RIGHT) ballPosition.x += 2 ok
if IsKeyDown(KEY_LEFT) ballPosition.x -= 2 ok
if IsKeyDown(KEY_UP) ballPosition.y -= 2 ok
if IsKeyDown(KEY_DOWN) ballPosition.y += 2 ok
BeginDrawing()
ClearBackground(RAYWHITE)
DrawText("move the ball with arrow keys", 10, 10, 20, DARKGRAY)
DrawCircleV(ballPosition, 50, MAROON)
EndDrawing()
end
CloseWindow()
Screen Shot:
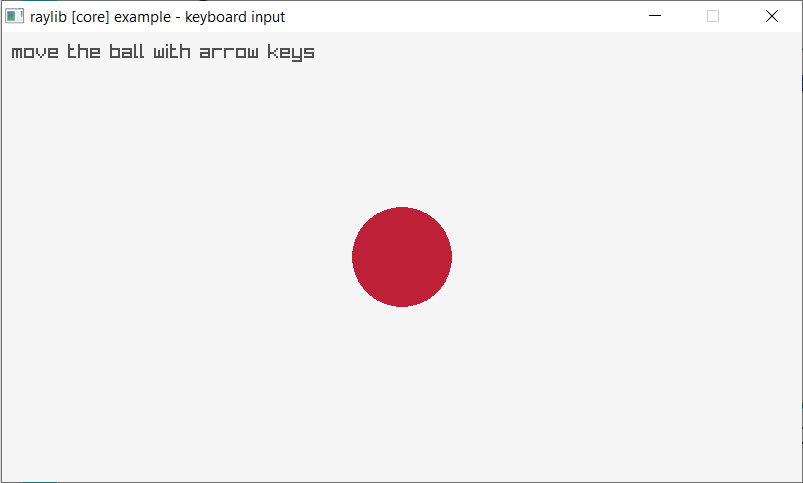
Input Mouse
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [core] example - mouse input")
ballPosition = Vector2(100, 100)
ballColor = DARKBLUE
SetTargetFPS(60)
while ! WindowShouldClose()
ballPosition = GetMousePosition()
if IsMouseButtonPressed(MOUSE_LEFT_BUTTON)
ballColor = MAROON
but IsMouseButtonPressed(MOUSE_MIDDLE_BUTTON)
ballColor = LIME
but IsMouseButtonPressed(MOUSE_RIGHT_BUTTON)
ballColor = DARKBLUE
ok
BeginDrawing()
ClearBackground(BLACK)
DrawCircleV(ballPosition, 40, ballColor)
DrawText("move ball with mouse and click mouse button to change color", 10, 10, 20, YELLOW)
EndDrawing()
end
CloseWindow()
Screen Shot:
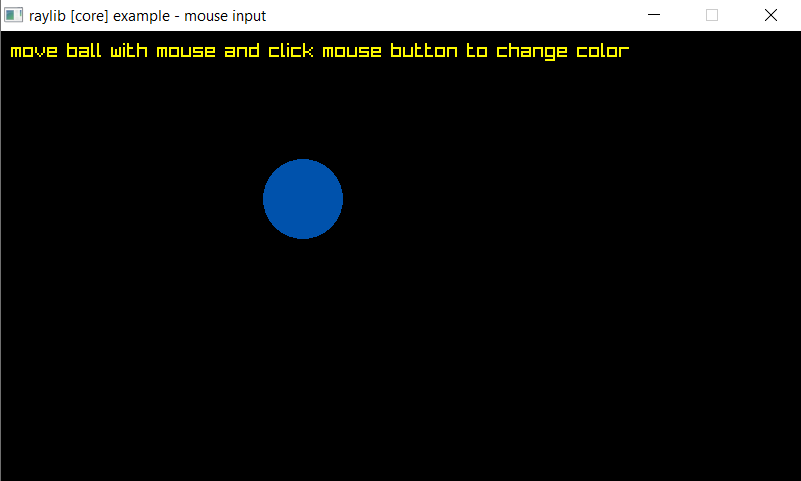
3D Camera
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [core] example - 3d camera mode")
camera = Camera3D(
0, 10, 10, // Camera position
0, 0, 0 , // Camera looking at point
0, 1, 0, // Camera up vector (rotation towards target)
45, // Camera field-of-view Y
CAMERA_PERSPECTIVE) // Camera mode type
cubePosition = Vector3(0, 0, 0)
SetTargetFPS(60)
while !WindowShouldClose()
BeginDrawing()
ClearBackground(RAYWHITE)
BeginMode3D(camera)
DrawCube(cubePosition, 2, 2, 2, RED)
DrawCubeWires(cubePosition, 2, 2, 2, MAROON)
DrawGrid(10, 1)
EndMode3D()
DrawText("Welcome to the third dimension!", 10, 40, 20, DARKGRAY)
DrawFPS(10, 10)
EndDrawing()
end
CloseWindow()
Screen Shot:
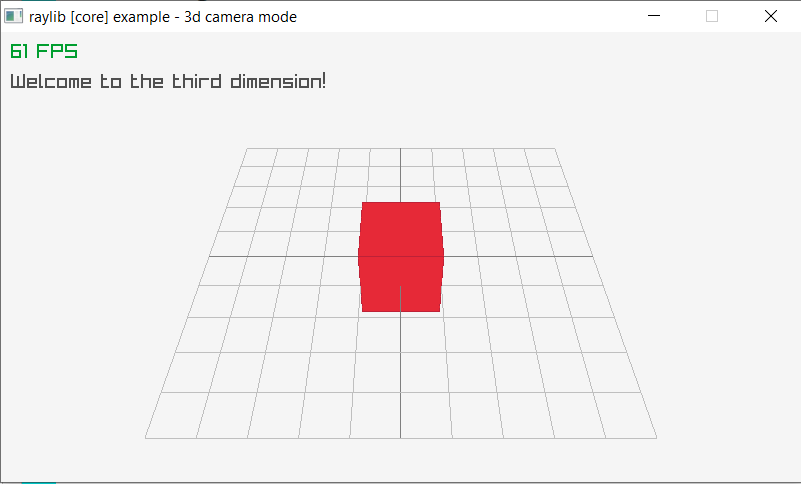
3D Camera Free
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [core] example - 3d camera free")
camera = Camera3D(
10, 10, 10, // Camera position
0, 0, 0 , // Camera looking at point
0, 1, 0, // Camera up vector (rotation towards target)
45, // Camera field-of-view Y
CAMERA_PERSPECTIVE) // Camera mode type
cubePosition = Vector3(0, 0, 0)
SetTargetFPS(60)
while !WindowShouldClose()
UpdateCamera(camera,CAMERA_FREE)
if IsKeyDown("Z") camera.target = Vector3( 0, 0, 0) ok
BeginDrawing()
ClearBackground(RAYWHITE)
BeginMode3D(camera)
DrawCube(cubePosition, 2, 2, 2, RED)
DrawCubeWires(cubePosition, 2, 2, 2, MAROON)
DrawGrid(10, 1)
EndMode3D()
DrawRectangle( 10, 10, 320, 133, Fade(SKYBLUE, 0.5))
DrawRectangleLines( 10, 10, 320, 133, BLUE)
DrawText("Free camera default controls:", 20, 20, 10, BLACK)
DrawText("- Mouse Wheel to Zoom in-out", 40, 40, 10, DARKGRAY)
DrawText("- Mouse Wheel Pressed to Pan", 40, 60, 10, DARKGRAY)
DrawText("- Alt + Mouse Wheel Pressed to Rotate", 40, 80, 10, DARKGRAY)
DrawText("- Alt + Ctrl + Mouse Wheel Pressed for Smooth Zoom", 40, 100, 10, DARKGRAY)
DrawText("- Z to zoom to (0, 0, 0)", 40, 120, 10, DARKGRAY)
EndDrawing()
end
CloseWindow()
Screen Shot:
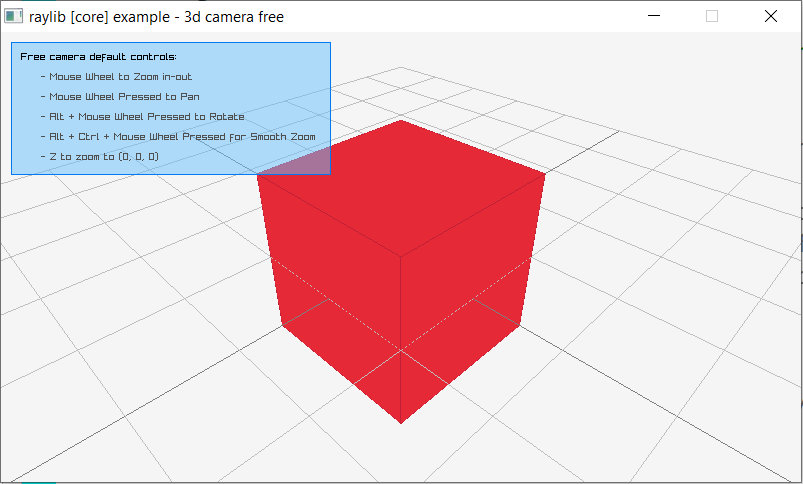
Mouse Wheel
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [core] example - input mouse wheel")
boxPositionY = screenHeight/2 - 40
scrollSpeed = 4
SetTargetFPS(60)
while !WindowShouldClose()
boxPositionY -= (GetMouseWheelMove()*scrollSpeed)
BeginDrawing()
ClearBackground(RAYWHITE)
DrawRectangle(screenWidth/2 - 40, boxPositionY, 80, 80, MAROON)
DrawText("Use mouse wheel to move the cube up and down!", 10, 10, 20, GRAY)
DrawText("Box position Y: "+boxPositionY, 10, 40, 20, LIGHTGRAY)
EndDrawing()
end
CloseWindow()
Screen Shot:
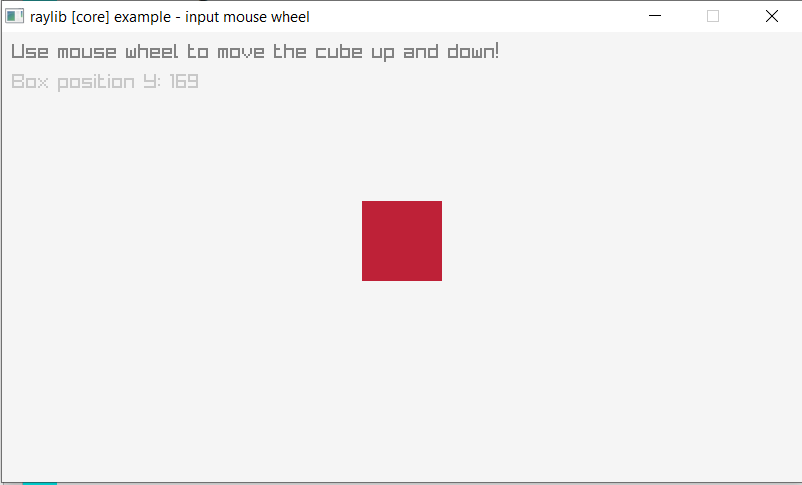
Input Multi-touch
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [core] example - input multitouch")
ballPosition = Vector2(-100, -100)
ballColor = BEIGE
touchCounter = 0
touchPosition = vector2(0,0)
MAX_TOUCH_POINTS = 5
SetTargetFPS(60)
while !WindowShouldClose()
ballPosition = GetMousePosition()
ballColor = BEIGE
if IsMouseButtonDown(MOUSE_LEFT_BUTTON) ballColor = MAROON ok
if IsMouseButtonDown(MOUSE_MIDDLE_BUTTON) ballColor = LIME ok
if IsMouseButtonDown(MOUSE_RIGHT_BUTTON) ballColor = DARKBLUE ok
if IsMouseButtonPressed(MOUSE_LEFT_BUTTON) touchCounter = 10 ok
if IsMouseButtonPressed(MOUSE_MIDDLE_BUTTON) touchCounter = 10 ok
if IsMouseButtonPressed(MOUSE_RIGHT_BUTTON) touchCounter = 10 ok
if touchCounter > 0 touchCounter-- ok
BeginDrawing()
ClearBackground(RAYWHITE)
for i = 0 to MAX_TOUCH_POINTS-1
touchPosition = GetTouchPosition(i)
if touchPosition.x >= 0 && touchPosition.y >= 0
DrawCircleV(touchPosition, 34, ORANGE)
DrawText(""+ i, touchPosition.x - 10, touchPosition.y - 70, 40, BLACK)
ok
next
DrawCircleV(ballPosition, 30 + (touchCounter*3), ballColor)
DrawText("move ball with mouse and click mouse button to change color", 10, 10, 20, DARKGRAY)
DrawText("touch the screen at multiple locations to get multiple balls", 10, 30, 20, DARKGRAY)
EndDrawing()
end
CloseWindow()
Screen Shot:
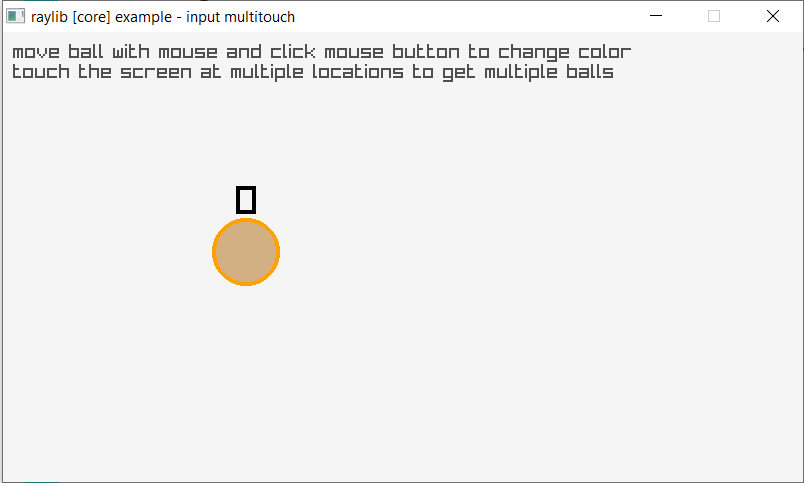
Camera First Person
load "raylib.ring"
MAX_COLUMNS = 20
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [core] example - 3d camera first person")
camera = Camera3d(
4, 2, 4,
0, 1, 0,
0, 1, 0,
60,
CAMERA_PERSPECTIVE
)
heights = list(MAX_COLUMNS)
positions = list(MAX_COLUMNS)
for item in positions item = vector3(0,0,0) next
colors = list(MAX_COLUMNS)
for item in colors item = BLACK next
for i = 1 to MAX_COLUMNS
heights[i] = GetRandomValue(1, 12)
positions[i] = Vector3(GetRandomValue(-15, 15), heights[i]/2, GetRandomValue(-15, 15) )
colors[i] = RAYLibColor(GetRandomValue(20, 255), GetRandomValue(10, 55), 30, 255 )
next
SetTargetFPS(60)
while !WindowShouldClose()
UpdateCamera(camera,CAMERA_FIRST_PERSON)
BeginDrawing()
ClearBackground(RAYWHITE)
BeginMode3D(camera)
DrawPlane(Vector3( 0, 0, 0 ), Vector2(32, 32 ), LIGHTGRAY) // Draw ground
DrawCube(Vector3( -16, 2.5, 0 ), 1, 5, 32, BLUE) // Draw a blue wall
DrawCube(Vector3( 16, 2.5, 0 ), 1, 5, 32, LIME) // Draw a green wall
DrawCube(Vector3( 0, 2.5, 16 ), 32, 5, 1, GOLD) // Draw a yellow wall
for i = 1 to MAX_COLUMNS
DrawCube(positions[i], 2, heights[i], 2, colors[i])
DrawCubeWires(positions[i], 2, heights[i], 2, MAROON)
next
EndMode3D()
DrawRectangle( 10, 10, 220, 70, Fade(SKYBLUE, 0.5f))
DrawRectangleLines( 10, 10, 220, 70, BLUE)
DrawText("First person camera default controls:", 20, 20, 10, BLACK)
DrawText("- Move with keys: W, A, S, D", 40, 40, 10, DARKGRAY)
DrawText("- Mouse move to look around", 40, 60, 10, DARKGRAY)
EndDrawing()
end
CloseWindow()
Screen Shot:
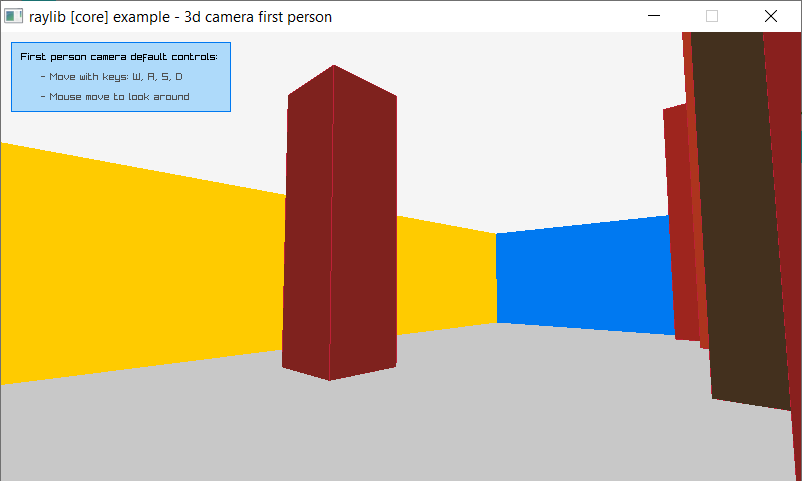
3D Picking
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [core] example - 3d picking")
camera = Camera3D(
10, 10, 10,
0, 0, 0 ,
0, 1, 0 ,
45,
CAMERA_PERSPECTIVE
)
cubePosition = Vector3( 0, 1, 0 )
cubeSize = Vector3( 2, 2, 2 )
ray = Ray(0,0,0,0,0,0)
collision = false
SetTargetFPS(60)
while !WindowShouldClose()
if IsMouseButtonPressed(MOUSE_LEFT_BUTTON)
if !collision
ray = GetMouseRay(GetMousePosition(), camera)
collision = GetRayCollisionBox(ray,
BoundingBox( cubePosition.x - cubeSize.x/2, cubePosition.y - cubeSize.y/2, cubePosition.z - cubeSize.z/2,
cubePosition.x + cubeSize.x/2, cubePosition.y + cubeSize.y/2, cubePosition.z + cubeSize.z/2 ) )
collision = collision.hit
else collision = false
ok
ok
BeginDrawing()
ClearBackground(RAYWHITE)
BeginMode3D(camera)
if collision
DrawCube(cubePosition, cubeSize.x, cubeSize.y, cubeSize.z, RED)
DrawCubeWires(cubePosition, cubeSize.x, cubeSize.y, cubeSize.z, MAROON)
DrawCubeWires(cubePosition, cubeSize.x + 0.2f, cubeSize.y + 0.2f, cubeSize.z + 0.2f, GREEN)
else
DrawCube(cubePosition, cubeSize.x, cubeSize.y, cubeSize.z, GRAY)
DrawCubeWires(cubePosition, cubeSize.x, cubeSize.y, cubeSize.z, DARKGRAY)
ok
DrawRay(ray, MAROON)
DrawGrid(10, 1)
EndMode3D()
DrawText("Try selecting the box with mouse!", 240, 10, 20, DARKGRAY)
if collision DrawText("BOX SELECTED", (screenWidth - MeasureText("BOX SELECTED", 30)) / 2, screenHeight * 0.1f, 30, GREEN) ok
DrawFPS(10, 10)
EndDrawing()
end
CloseWindow()
Screen Shot:
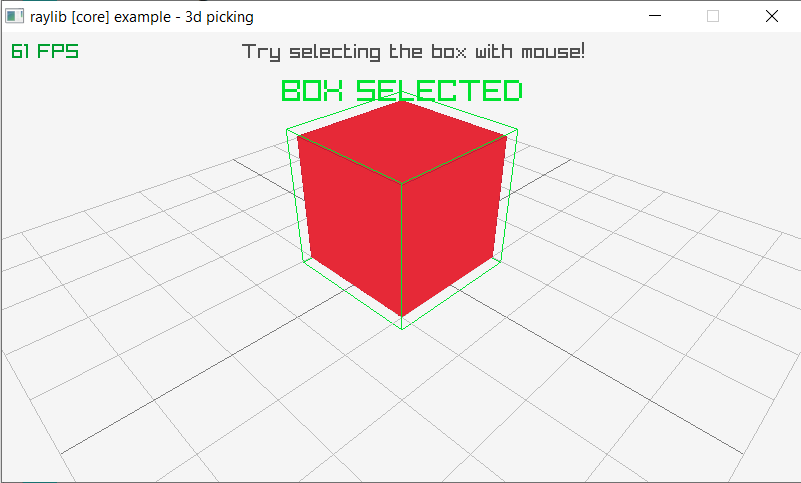
Full Screen
load "raylib.ring"
screenWidth = 1024
screenHeight = 768
InitWindow(screenWidth, screenHeight, "Full Screen")
ToggleFullScreen()
SetTargetFPS(60)
while !WindowShouldClose()
BeginDrawing()
ClearBackground(DARKBLUE)
DrawText("Count from 1 to 10", 190, 200, 20, Yellow)
for t = 1 to 10
DrawText("Number: " + t, 190, 200+(30*t), 20, WHITE)
next
EndDrawing()
end
CloseWindow()
Screen Shot:
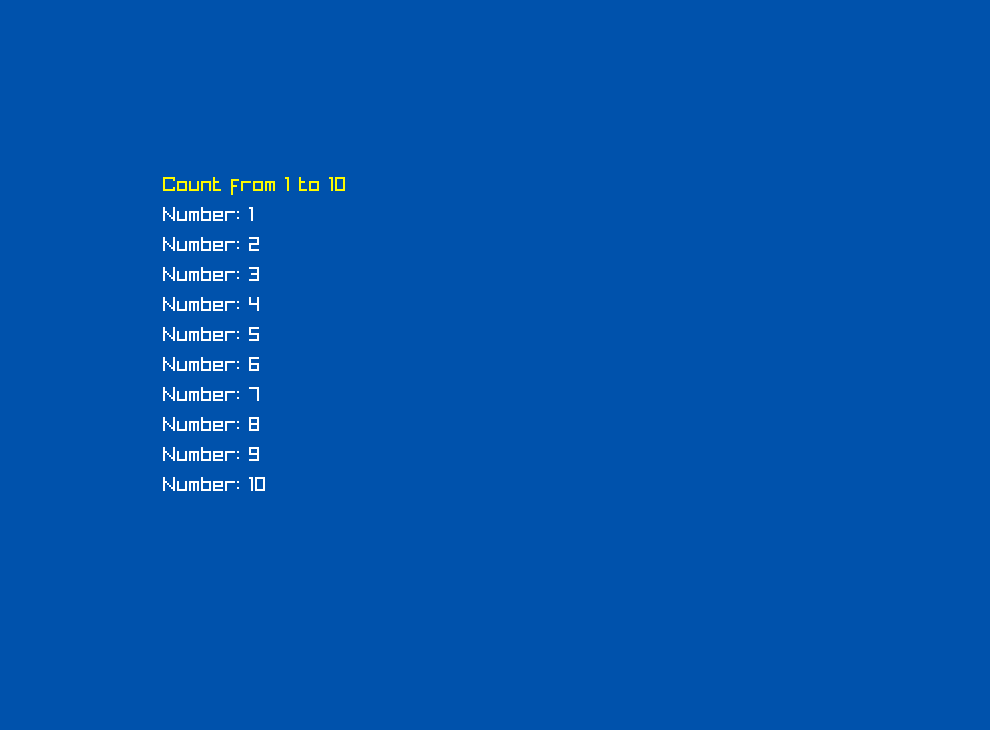
Two Cubes
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [core] example - Two Cubes")
camera = Camera3D(
10, 10, 10,
0, 0, 0 ,
0, 1, 0 ,
45,
CAMERA_PERSPECTIVE
)
cubePosition1 = Vector3( 0, 1, 4 )
cubePosition2 = Vector3( 0, 1, -4 )
cubeSize = Vector3( 2, 2, 2 )
ray = Ray(0,0,0,0,0,0)
collision1 = false
collision2 = false
SetTargetFPS(60)
while !WindowShouldClose()
if IsMouseButtonPressed(MOUSE_LEFT_BUTTON)
if !collision1
ray = GetMouseRay(GetMousePosition(), camera)
collision1 = GetRayCollisionBox(ray,
BoundingBox( cubePosition1.x - cubeSize.x/2, cubePosition1.y - cubeSize.y/2, cubePosition1.z - cubeSize.z/2,
cubePosition1.x + cubeSize.x/2, cubePosition1.y + cubeSize.y/2, cubePosition1.z + cubeSize.z/2 ) )
collision1 = collision1.hit
else
collision1 = false
ok
if !collision2
ray = GetMouseRay(GetMousePosition(), camera)
collision2 = GetRayCollisionBox(ray,
BoundingBox( cubePosition2.x - cubeSize.x/2, cubePosition2.y - cubeSize.y/2, cubePosition2.z - cubeSize.z/2,
cubePosition2.x + cubeSize.x/2, cubePosition2.y + cubeSize.y/2, cubePosition2.z + cubeSize.z/2 ) )
collision2 = collision2.hit
else
collision2 = false
ok
ok
BeginDrawing()
ClearBackground(RAYWHITE)
BeginMode3D(camera)
if collision1
DrawCube(cubePosition1, cubeSize.x, cubeSize.y, cubeSize.z, RED)
DrawCubeWires(cubePosition1, cubeSize.x, cubeSize.y, cubeSize.z, MAROON)
DrawCubeWires(cubePosition1, cubeSize.x + 0.2f, cubeSize.y + 0.2f, cubeSize.z + 0.2f, GREEN)
collision1 = true
else
DrawCube(cubePosition1, cubeSize.x, cubeSize.y, cubeSize.z, GRAY)
DrawCubeWires(cubePosition1, cubeSize.x, cubeSize.y, cubeSize.z, DARKGRAY)
collision1 = false
ok
if collision2
DrawCube(cubePosition2, cubeSize.x, cubeSize.y, cubeSize.z, RED)
DrawCubeWires(cubePosition2, cubeSize.x, cubeSize.y, cubeSize.z, MAROON)
DrawCubeWires(cubePosition2, cubeSize.x + 0.2f, cubeSize.y + 0.2f, cubeSize.z + 0.2f, GREEN)
collision2 = true
else
DrawCube(cubePosition2, cubeSize.x, cubeSize.y, cubeSize.z, GRAY)
DrawCubeWires(cubePosition2, cubeSize.x, cubeSize.y, cubeSize.z, DARKGRAY)
collision2 = false
ok
DrawRay(ray, MAROON)
DrawGrid(10, 1)
EndMode3D()
DrawText("Try selecting the box with mouse!", 240, 10, 20, DARKGRAY)
if collision1 or collision2 DrawText("BOX SELECTED", (screenWidth - MeasureText("BOX SELECTED", 30)) / 2, screenHeight * 0.1f, 30, GREEN) ok
DrawFPS(10, 10)
EndDrawing()
end
CloseWindow()
Screen Shot:
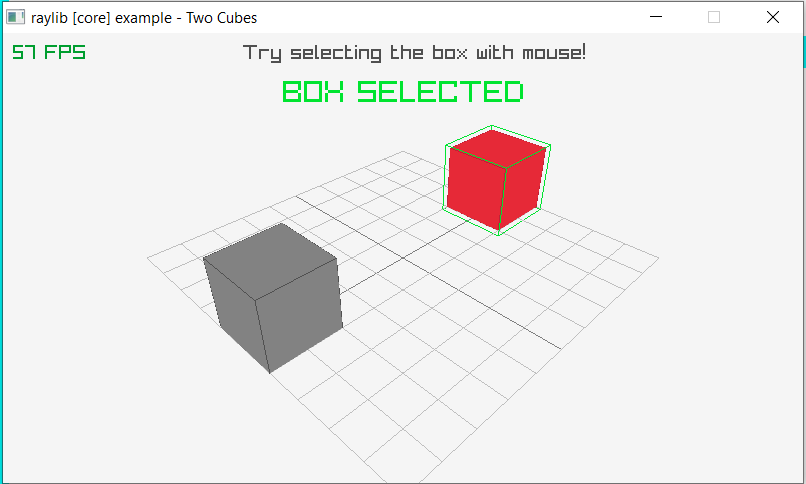
Basic Shapes
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [shapes] example - basic shapes drawing")
SetTargetFPS(60)
while !WindowShouldClose()
BeginDrawing()
ClearBackground(RAYWHITE)
DrawText("some basic shapes available on raylib", 20, 20, 20, DARKGRAY)
DrawCircle(screenWidth/4, 120, 35, DARKBLUE)
DrawRectangle(screenWidth/4*2 - 60, 100, 120, 60, RED)
DrawRectangleLines(screenWidth/4*2 - 40, 320, 80, 60, ORANGE)
DrawRectangleGradientH(screenWidth/4*2 - 90, 170, 180, 130, MAROON, GOLD)
DrawTriangle(Vector2(screenWidth/4*3, 80),
Vector2(screenWidth/4*3 - 60, 150),
Vector2(screenWidth/4*3 + 60, 150), VIOLET)
DrawPoly(Vector2(screenWidth/4*3, 320), 6, 80, 0, BROWN)
DrawCircleGradient(screenWidth/4, 220, 60, GREEN, SKYBLUE)
DrawLine(18, 42, screenWidth - 18, 42, BLACK)
DrawCircleLines(screenWidth/4, 340, 80, DARKBLUE)
DrawTriangleLines(Vector2(screenWidth/4*3, 160),
Vector2(screenWidth/4*3 - 20, 230),
Vector2(screenWidth/4*3 + 20, 230), DARKBLUE)
EndDrawing()
end
CloseWindow()
Screen Shot:
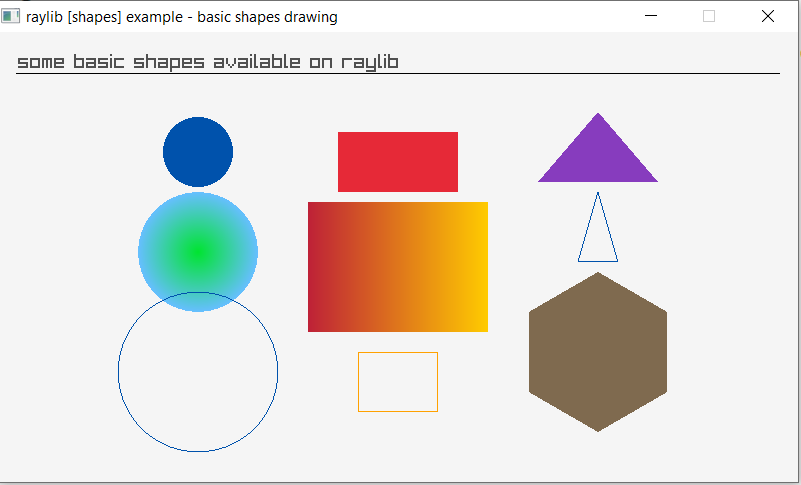
Draw Ring
load "raylib.ring"
screenWidth = 800 screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [shapes] example - draw ring")
center = Vector2((GetScreenWidth() - 300)/2, GetScreenHeight()/2 )
innerRadius = 80 outerRadius = 190
startAngle = 0 endAngle = 360 segments = 0
drawRing = true drawRingLines = false drawCircleLines = false
SetTargetFPS(60)
while !WindowShouldClose()
BeginDrawing()
ClearBackground(RAYWHITE)
DrawLine(500, 0, 500, GetScreenHeight(), Fade(LIGHTGRAY, 0.6))
DrawRectangle(500, 0, GetScreenWidth() - 500, GetScreenHeight(), Fade(LIGHTGRAY, 0.3))
if drawRing DrawRing(center, innerRadius, outerRadius, startAngle, endAngle, segments, Fade(MAROON, 0.3)) ok
if drawRingLines DrawRingLines(center, innerRadius, outerRadius, startAngle, endAngle, segments, Fade(BLACK, 0.4)) ok
if drawCircleLines DrawCircleSectorLines(center, outerRadius, startAngle, endAngle, segments, Fade(BLACK, 0.4)) ok
startAngle = GuiSliderBar(Rectangle( 600, 40, 120, 20 ), "StartAngle", startAngle, -450, 450, true)
endAngle = GuiSliderBar(Rectangle( 600, 70, 120, 20 ), "EndAngle", endAngle, -450, 450, true)
innerRadius = GuiSliderBar(Rectangle( 600, 140, 120, 20 ), "InnerRadius", innerRadius, 0, 100, true)
outerRadius = GuiSliderBar(Rectangle( 600, 170, 120, 20 ), "OuterRadius", outerRadius, 0, 200, true)
segments = GuiSliderBar(Rectangle( 600, 240, 120, 20 ), "Segments", segments, 0, 100, true)
drawRing = GuiCheckBox(Rectangle( 600, 320, 20, 20 ), "Draw Ring", drawRing)
drawRingLines = GuiCheckBox(Rectangle( 600, 350, 20, 20 ), "Draw RingLines", drawRingLines)
drawCircleLines = GuiCheckBox(Rectangle( 600, 380, 20, 20 ), "Draw CircleLines", drawCircleLines)
if segments >= 4 DrawText("MODE: MANUAL", 600, 270, 10, MAROON)
else DrawText("MODE: AUTO", 600, 270, 10, DARKGRAY) ok
DrawFPS(10, 10)
EndDrawing()
end
CloseWindow()
Screen Shot:
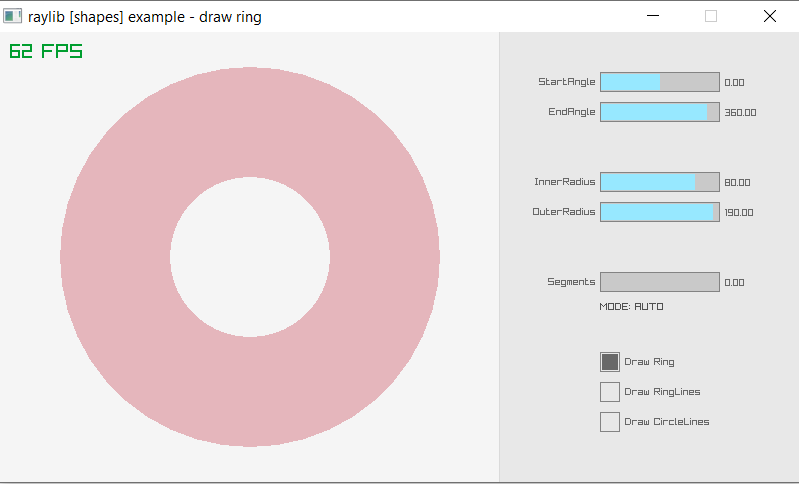
Screen Shot (2):
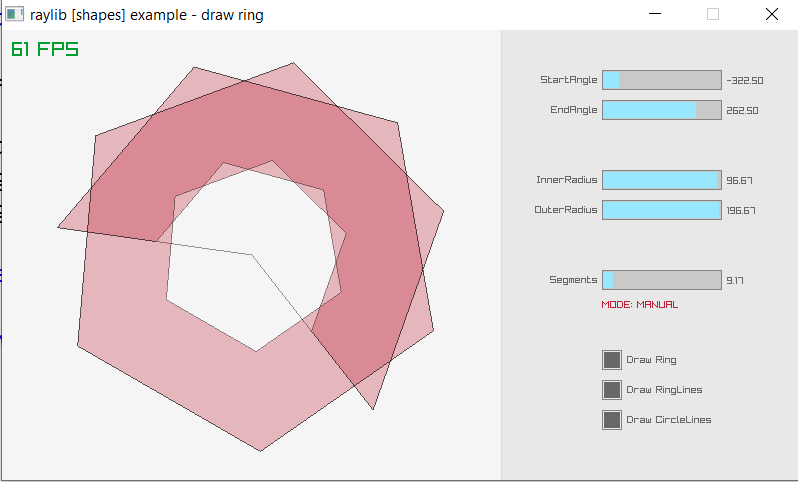
Bezier Lines
load "raylib.ring"
screenWidth = 800
screenHeight = 450
SetConfigFlags(FLAG_MSAA_4X_HINT)
InitWindow(screenWidth, screenHeight, "raylib [shapes] example - cubic-bezier lines")
start = Vector2(0,0)
endvec = Vector2(screenWidth,screenHeight)
SetTargetFPS(60)
while (!WindowShouldClose())
if (IsMouseButtonDown(MOUSE_LEFT_BUTTON))
start = GetMousePosition()
else (IsMouseButtonDown(MOUSE_RIGHT_BUTTON))
endvec = GetMousePosition()
ok
BeginDrawing()
ClearBackground(RAYWHITE)
DrawText("USE MOUSE LEFT-RIGHT CLICK to DEFINE LINE START and END POINTS", 15, 20, 20, GRAY)
DrawLineBezier(start, endvec, 2.0, RED)
EndDrawing()
end
CloseWindow()
Screen Shot:
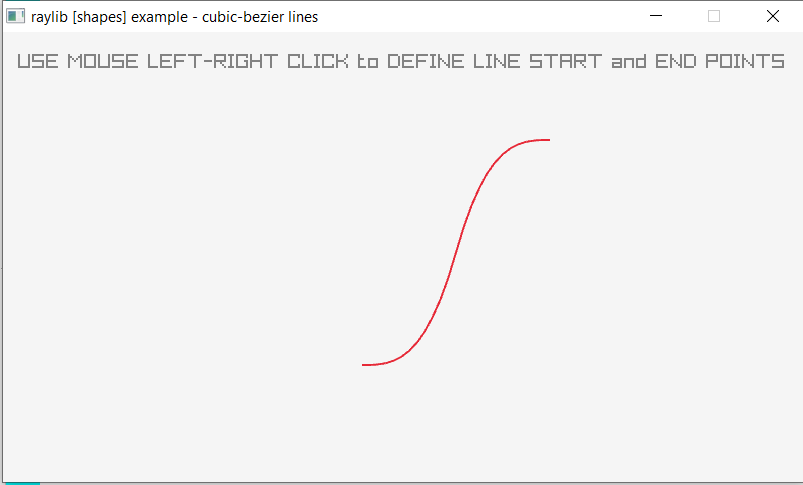
Collision Area
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [shapes] example - collision area")
// Box A: Moving box
boxA = Rectangle( 10, GetScreenHeight()/2 - 50, 200, 100 )
boxASpeedX = 4
// Box B: Mouse moved box
boxB = Rectangle( GetScreenWidth()/2 - 30, GetScreenHeight()/2 - 30, 60, 60 )
boxCollision = GetCollisionRec(boxA, boxB)
boxCollision = Rectangle( 0,0,0,0 ) // Collision rectangle
screenUpperLimit = 40 // Top menu limits
pause = false // Movement pause
collision = false // Collision detection
SetTargetFPS(60)
while !WindowShouldClose()
// Move box if not paused
if (not pause) boxA.x += boxASpeedX ok
// Bounce box on x screen limits
if (((boxA.x + boxA.width) >= GetScreenWidth()) or (boxA.x <= 0)) boxASpeedX = boxASpeedX*(-1) ok
// Update player-controlled-box (box02)
boxB.x = GetMouseX() - boxB.width/2
boxB.y = GetMouseY() - boxB.height/2
// Make sure Box B does not go out of move area limits
if ((boxB.x + boxB.width) >= GetScreenWidth()) boxB.x = GetScreenWidth() - boxB.width
else (boxB.x <= 0) boxB.x = 0 ok
if ((boxB.y + boxB.height) >= GetScreenHeight()) boxB.y = GetScreenHeight() - boxB.height
else (boxB.y <= screenUpperLimit) boxB.y = screenUpperLimit ok
// Check boxes collision
collision = CheckCollisionRecs(boxA, boxB)
// Get collision rectangle (only on collision)
if (collision) boxCollision = GetCollisionRec(boxA, boxB) ok
// Pause Box A movement
if (IsKeyPressed(KEY_SPACE)) pause = not pause ok
BeginDrawing()
ClearBackground(RAYWHITE)
if collision = true
color = RED
else
color = BLACK
ok
DrawRectangle(0, 0, screenWidth, screenUpperLimit, color)
DrawRectangleRec(boxA, GOLD)
boxB.x = GetMouseX() - boxB.width/2
boxB.y = GetMouseY() - boxB.height/2
collision = CheckCollisionRecs(boxA, boxB)
DrawRectangleRec(boxB, BLUE)
boxCollision = GetCollisionRec(boxA, boxB)
if (collision) = true
// Draw collision area
DrawRectangleRec(boxCollision, LIME)
// Draw collision message
DrawText("COLLISION!", GetScreenWidth()/2 - MeasureText("COLLISION!", 20)/2, screenUpperLimit/2 - 10, 20, BLACK)
// Draw collision area
DrawText("Collision Area: " + string(boxCollision.width*boxCollision.height), GetScreenWidth()/2 - 100, screenUpperLimit + 10, 20, BLACK)
ok
DrawFPS(10, 10)
EndDrawing()
end
CloseWindow()
Screen Shot:
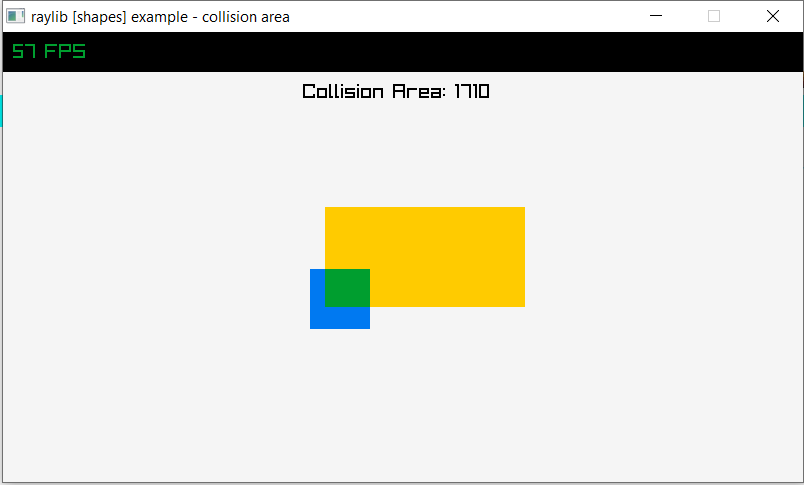
Following Eyes
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [shapes] example - following eyes")
scleraLeftPosition = Vector2( GetScreenWidth()/2 - 100, GetScreenHeight()/2 )
scleraRightPosition = Vector2( GetScreenWidth()/2 + 100, GetScreenHeight()/2 )
scleraRadius = 80
irisLeftPosition = Vector2( GetScreenWidth()/2 - 100, GetScreenHeight()/2 )
irisRightPosition = Vector2( GetScreenWidth()/2 + 100, GetScreenHeight()/2 )
irisRadius = 24
angle = 0.0
dx = 0.0 dy = 0.0 dxx = 0.0 dyy = 0.0
SetTargetFPS(60)
while !WindowShouldClose()
irisLeftPosition = GetMousePosition()
irisRightPosition = GetMousePosition()
// Check not inside the left eye sclera
if !CheckCollisionPointCircle(irisLeftPosition, scleraLeftPosition, scleraRadius - 20)
dx = irisLeftPosition.x - scleraLeftPosition.x
dy = irisLeftPosition.y - scleraLeftPosition.y
angle = atan2(dy, dx)
dxx = (scleraRadius - irisRadius)*cos(angle)
dyy = (scleraRadius - irisRadius)*sin(angle)
irisLeftPosition.x = scleraLeftPosition.x + dxx
irisLeftPosition.y = scleraLeftPosition.y + dyy
ok
// Check not inside the right eye sclera
if !CheckCollisionPointCircle(irisRightPosition, scleraRightPosition, scleraRadius - 20)
dx = irisRightPosition.x - scleraRightPosition.x
dy = irisRightPosition.y - scleraRightPosition.y
angle = atan2(dy, dx)
dxx = (scleraRadius - irisRadius)*cos(angle)
dyy = (scleraRadius - irisRadius)*sin(angle)
irisRightPosition.x = scleraRightPosition.x + dxx
irisRightPosition.y = scleraRightPosition.y + dyy
ok
BeginDrawing()
ClearBackground(RAYWHITE)
DrawCircleV(scleraLeftPosition, scleraRadius, LIGHTGRAY)
DrawCircleV(irisLeftPosition, irisRadius, BROWN)
DrawCircleV(irisLeftPosition, 10, BLACK)
DrawCircleV(scleraRightPosition, scleraRadius, LIGHTGRAY)
DrawCircleV(irisRightPosition, irisRadius, DARKGREEN)
DrawCircleV(irisRightPosition, 10, BLACK)
DrawFPS(10, 10)
EndDrawing()
end
CloseWindow()
Screen Shot:
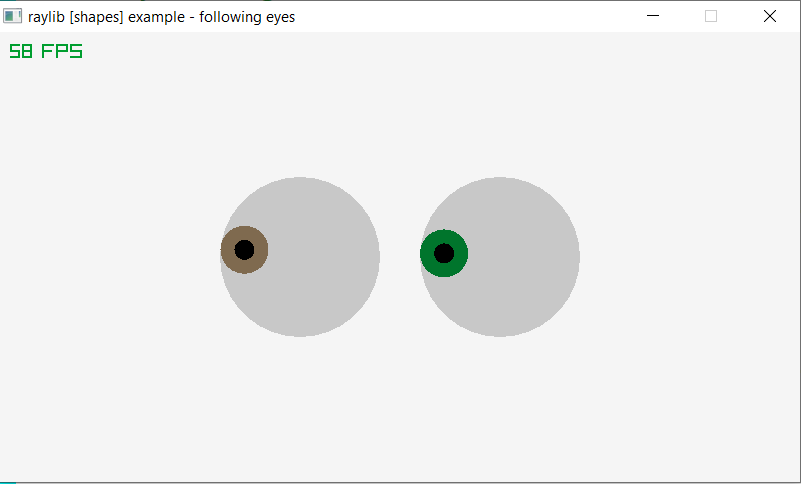
Colors Palette
load "raylib.ring"
MAX_COLORS_COUNT = 21 // Number of colors available
screenWidth = 800
screenHeight = 450
colors = list(MAX_COLORS_COUNT)
colorNames = list(MAX_COLORS_COUNT)
colorsRecs = list(MAX_COLORS_COUNT)
colorState = list(MAX_COLORS_COUNT)
InitWindow(screenWidth, screenHeight, "raylib [shapes] example - colors palette")
colors = [
DARKGRAY, MAROON, ORANGE, DARKGREEN, DARKBLUE, DARKPURPLE, DARKBROWN,
GRAY, RED, GOLD, LIME, BLUE, VIOLET, BROWN, LIGHTGRAY, PINK, YELLOW,
GREEN, SKYBLUE, PURPLE, BEIGE ]
colorNames = [
"DARKGRAY", "MAROON", "ORANGE", "DARKGREEN", "DARKBLUE", "DARKPURPLE",
"DARKBROWN", "GRAY", "RED", "GOLD", "LIME", "BLUE", "VIOLET", "BROWN",
"LIGHTGRAY", "PINK", "YELLOW", "GREEN", "SKYBLUE", "PURPLE", "BEIGE" ]
for i = 1 to MAX_COLORS_COUNT
colorsRecs[i] = new Rectangle(0,0,0,0)
next
for i = 1 to MAX_COLORS_COUNT
colorState[i] = 0
next
// Fills colorsRecs data (for every rectangle)
for i = 1 to MAX_COLORS_COUNT
colorsRecs[i].x = 20 + 100*((i-1)%7) + 10*((i-1)%7)
colorsRecs[i].y = 80 + 100*floor((i-1)/7) + 10*floor((i-1)/7)
colorsRecs[i].width = 100
colorsRecs[i].height = 100
next
mousePoint = Vector2( 0.0, 0.0 )
SetTargetFPS(60)
// Main game loop
while !WindowShouldClose()
mousePoint = GetMousePosition()
for i = 1 to MAX_COLORS_COUNT
if (CheckCollisionPointRec(mousePoint, colorsRecs[i])) colorState[i] = 1
else colorState[i] = 0 ok
next
BeginDrawing()
ClearBackground(RAYWHITE)
DrawText("raylib colors palette", 28, 42, 20, BLACK)
DrawText("press SPACE to see all colors", GetScreenWidth() - 180, GetScreenHeight() - 40, 10, GRAY)
for i = 1 to MAX_COLORS_COUNT // Draw all rectangles
if colorState[i]
cstate = 0.6
else
cstate = 1.0
ok
DrawRectangleRec(colorsRecs[i], Fade(colors[i], cstate))
if (IsKeyDown(KEY_SPACE) || colorState[i])
DrawRectangle(colorsRecs[i].x, colorsRecs[i].y + colorsRecs[i].height - 26, colorsRecs[i].width, 20, BLACK)
DrawRectangleLinesEx(colorsRecs[i], 6, Fade(BLACK, 0.3f))
DrawText(colorNames[i], colorsRecs[i].x + colorsRecs[i].width - MeasureText(colorNames[i], 10) - 12,
colorsRecs[i].y + colorsRecs[i].height - 20, 10, colors[i])
ok
next
EndDrawing()
end
CloseWindow()
Screen Shot:
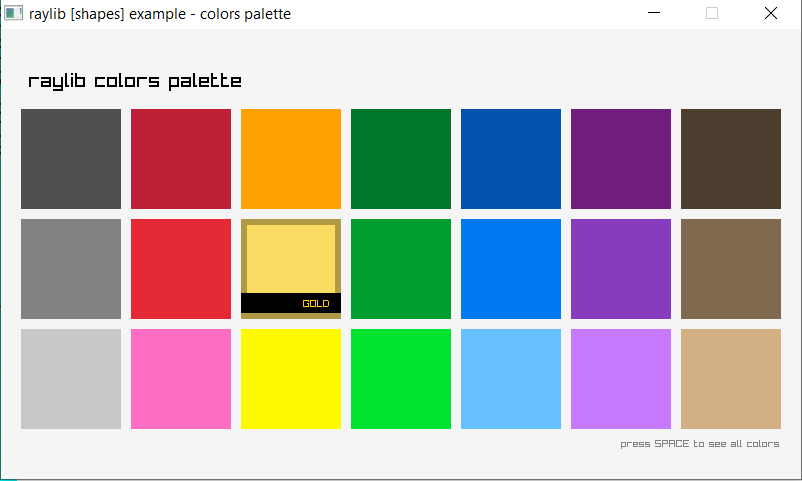
Rectangle Scaling
load "raylib.ring"
MOUSE_SCALE_MARK_SIZE = 12
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [shapes] example - rectangle scaling mouse")
rec = Rectangle( 100, 100, 200, 80 )
mousePosition = Vector2( 0,0 )
mouseScaleReady = false
mouseScaleMode = false
SetTargetFPS(60)
while !WindowShouldClose()
mousePosition = GetMousePosition()
if (CheckCollisionPointRec(mousePosition, rec) and
CheckCollisionPointRec(mousePosition, Rectangle(rec.x + rec.width - MOUSE_SCALE_MARK_SIZE, rec.y + rec.height - MOUSE_SCALE_MARK_SIZE, MOUSE_SCALE_MARK_SIZE, MOUSE_SCALE_MARK_SIZE )))
mouseScaleReady = true
if (IsMouseButtonPressed(MOUSE_LEFT_BUTTON)) mouseScaleMode = true ok
else mouseScaleReady = false ok
if (mouseScaleMode)
mouseScaleReady = true
rec.width = (mousePosition.x - rec.x)
rec.height = (mousePosition.y - rec.y)
if (rec.width < MOUSE_SCALE_MARK_SIZE) rec.width = MOUSE_SCALE_MARK_SIZE ok
if (rec.height < MOUSE_SCALE_MARK_SIZE) rec.height = MOUSE_SCALE_MARK_SIZE ok
if (IsMouseButtonReleased(MOUSE_LEFT_BUTTON)) mouseScaleMode = false ok
ok
BeginDrawing()
ClearBackground(RAYWHITE)
DrawText("Scale rectangle dragging from bottom-right corner!", 10, 10, 20, GRAY)
DrawRectangleRec(rec, Fade(GREEN, 0.5f))
if (mouseScaleReady)
DrawRectangleLinesEx(rec, 1, RED)
DrawTriangle(Vector2( rec.x + rec.width - MOUSE_SCALE_MARK_SIZE, rec.y + rec.height ),
Vector2( rec.x + rec.width, rec.y + rec.height ),
Vector2( rec.x + rec.width, rec.y + rec.height - MOUSE_SCALE_MARK_SIZE ), RED)
ok
EndDrawing()
end
CloseWindow()
Screen Shot:
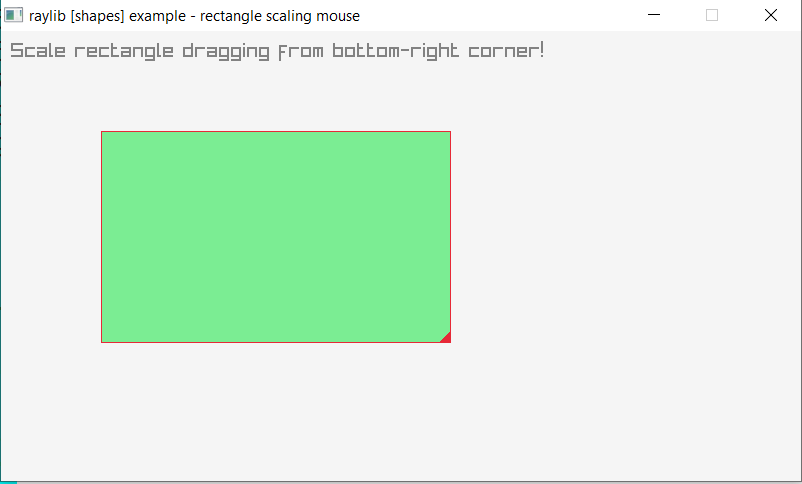
Music Playing
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [audio] example - music playing (streaming)")
InitAudioDevice()
music = LoadMusicStream("guitar_noodling.ogg")
PlayMusicStream(music)
timePlayed = 0.0
pause = false
SetTargetFPS(60)
while !WindowShouldClose()
UpdateMusicStream(music)
if IsKeyPressed(KEY_SPACE)
StopMusicStream(music)
PlayMusicStream(music)
ok
if IsKeyPressed(KEY_P)
pause = !pause
if pause
PauseMusicStream(music)
else
ResumeMusicStream(music)
ok
ok
timePlayed = GetMusicTimePlayed(music) / GetMusicTimeLength(music) *400
if timePlayed > 400
StopMusicStream(music)
ok
BeginDrawing()
ClearBackground(RAYWHITE)
DrawText("MUSIC SHOULD BE PLAYING!", 255, 150, 20, LIGHTGRAY)
DrawRectangle(200, 200, 400, 12, LIGHTGRAY)
DrawRectangle(200, 200, timePlayed, 12, MAROON)
DrawRectangleLines(200, 200, 400, 12, GRAY)
DrawText("PRESS SPACE TO RESTART MUSIC", 215, 250, 20, LIGHTGRAY)
DrawText("PRESS P TO PAUSE/RESUME MUSIC", 208, 280, 20, LIGHTGRAY)
EndDrawing()
end
UnloadMusicStream(music)
CloseAudioDevice()
CloseWindow()
Screen Shot:
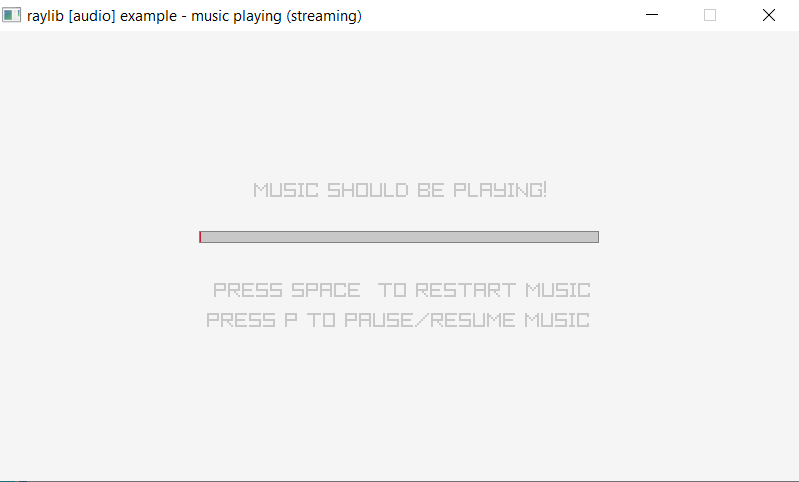
Sound Loading
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [audio] example - sound loading and playing")
InitAudioDevice()
fxWav = LoadSound("sound.wav")
fxOgg = LoadSound("tanatana.ogg")
SetTargetFPS(60)
while !WindowShouldClose()
if IsKeyPressed(KEY_SPACE) PlaySound(fxWav) ok
if IsKeyPressed(KEY_ENTER) PlaySound(fxOgg) ok
BeginDrawing()
ClearBackground(RAYWHITE)
DrawText("Press SPACE to PLAY the WAV sound!", 200, 180, 20, LIGHTGRAY)
DrawText("Press ENTER to PLAY the OGG sound!", 200, 220, 20, LIGHTGRAY)
EndDrawing()
end
UnloadSound(fxWav)
UnloadSound(fxOgg)
CloseAudioDevice()
CloseWindow()
Screen Shot:
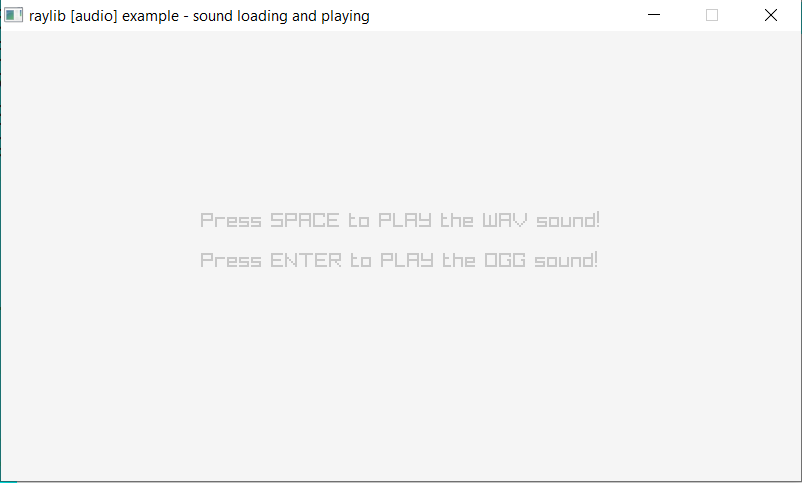
Image Drawing
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [textures] example - image drawing")
cat = LoadImage("cat.png")
ImageCrop( cat, Rectangle( 100, 10, 280, 380 ))
ImageFlipHorizontal( cat)
ImageResize( cat, 150, 200)
parrots = LoadImage("parrots.png")
ImageDraw( parrots, cat, Rectangle( 0, 0, cat.width, cat.height ), Rectangle( 30, 40, cat.width*1.5, cat.height*1.5 ))
ImageCrop( parrots, Rectangle( 0, 50, parrots.width, parrots.height - 100 ))
UnloadImage(cat)
font = LoadFont("custom_jupiter_crash.png")
ImageDrawTextEx(parrots, Vector2( 300, 230 ), font, "PARROTS & CAT", font.baseSize, -2, WHITE)
UnloadFont(font);
texture = LoadTextureFromImage(parrots)
UnloadImage(parrots)
SetTargetFPS(60)
while !WindowShouldClose()
BeginDrawing()
ClearBackground(RAYWHITE)
DrawTexture(texture, screenWidth/2 - texture.width/2, screenHeight/2 - texture.height/2 - 40, WHITE)
DrawRectangleLines(screenWidth/2 - texture.width/2, screenHeight/2 - texture.height/2 - 40, texture.width, texture.height, DARKGRAY)
DrawText("We are drawing only one texture from various images composed!", 240, 350, 10, DARKGRAY)
DrawText("Source images have been cropped, scaled, flipped and copied one over the other.", 190, 370, 10, DARKGRAY)
EndDrawing()
end
UnloadTexture(texture)
CloseWindow()
Screen Shot:
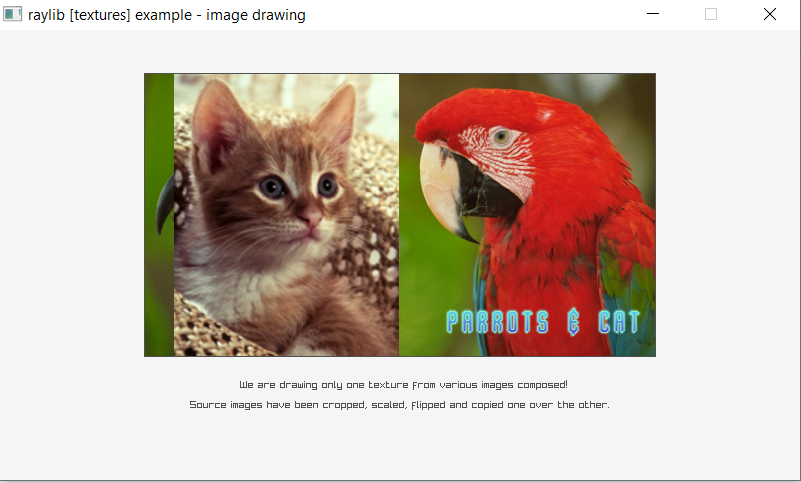
Image Generation
load "raylib.ring"
NUM_TEXTURES = 9
textures = list(NUM_TEXTURES)
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [textures] example - procedural images generation")
verticalGradient = GenImageGradientLinear(screenWidth, screenHeight, 0, RED, BLUE);
horizontalGradient = GenImageGradientLinear(screenWidth, screenHeight, 90, RED, BLUE);
diagonalGradient = GenImageGradientLinear(screenWidth, screenHeight, 45, RED, BLUE);
radialGradient = GenImageGradientRadial(screenWidth, screenHeight, 0.0f, WHITE, BLACK);
squareGradient = GenImageGradientSquare(screenWidth, screenHeight, 0.0f, WHITE, BLACK);
checked = GenImageChecked(screenWidth, screenHeight, 32, 32, RED, BLUE);
whiteNoise = GenImageWhiteNoise(screenWidth, screenHeight, 0.5f);
perlinNoise = GenImagePerlinNoise(screenWidth, screenHeight, 50, 50, 4.0f);
cellular = GenImageCellular(screenWidth, screenHeight, 32);
textures[NUM_TEXTURES] = 0
textures[1] = LoadTextureFromImage(verticalGradient)
textures[2] = LoadTextureFromImage(horizontalGradient)
textures[3] = LoadTextureFromImage(diagonalGradient)
textures[4] = LoadTextureFromImage(radialGradient)
textures[5] = LoadTextureFromImage(squareGradient)
textures[6] = LoadTextureFromImage(checked)
textures[7] = LoadTextureFromImage(whiteNoise)
textures[8] = LoadTextureFromImage(perlinNoise)
textures[9] = LoadTextureFromImage(cellular)
UnloadImage(verticalGradient)
UnloadImage(horizontalGradient)
UnloadImage(diagonalGradient)
UnloadImage(radialGradient)
UnloadImage(squareGradient)
UnloadImage(checked)
UnloadImage(whiteNoise)
UnloadImage(perlinNoise)
UnloadImage(cellular)
currentTexture = 1
SetTargetFPS(10)
while !WindowShouldClose()
if IsMouseButtonPressed(MOUSE_LEFT_BUTTON) || IsKeyPressed(KEY_RIGHT)
currentTexture++
if currentTexture > NUM_TEXTURES currentTexture = 1 ok
ok
BeginDrawing()
ClearBackground(RAYWHITE)
DrawTexture(textures[currentTexture], 0, 0, WHITE)
DrawRectangle(30, 400, 325, 30, Fade(SKYBLUE, 0.5))
DrawRectangleLines(30, 400, 325, 30, Fade(WHITE, 0.5))
DrawText("MOUSE LEFT BUTTON to CYCLE PROCEDURAL TEXTURES", 40, 410, 10, WHITE)
switch(currentTexture)
on 1 DrawText("VERTICAL GRADIENT", 560, 10, 20, RAYWHITE)
on 2 DrawText("HORIZONTAL GRADIENT", 540, 10, 20, RAYWHITE)
on 3 DrawText("DIAGONAL GRADIENT", 540, 10, 20, RAYWHITE)
on 4 DrawText("RADIAL GRADIENT", 580, 10, 20, LIGHTGRAY)
on 5 DrawText("SQUARE GRADIENT", 580, 10, 20, LIGHTGRAY)
on 6 DrawText("CHECKED", 680, 10, 20, RAYWHITE)
on 7 DrawText("WHITE NOISE", 640, 10, 20, RED)
on 8 DrawText("PERLIN NOISE", 630, 10, 20, RAYWHITE)
on 9 DrawText("CELLULAR", 670, 10, 20, RAYWHITE)
off
EndDrawing()
end
for i = 1 to NUM_TEXTURES
UnloadTexture( textures[i] )
next
CloseWindow()
Screen Shot:
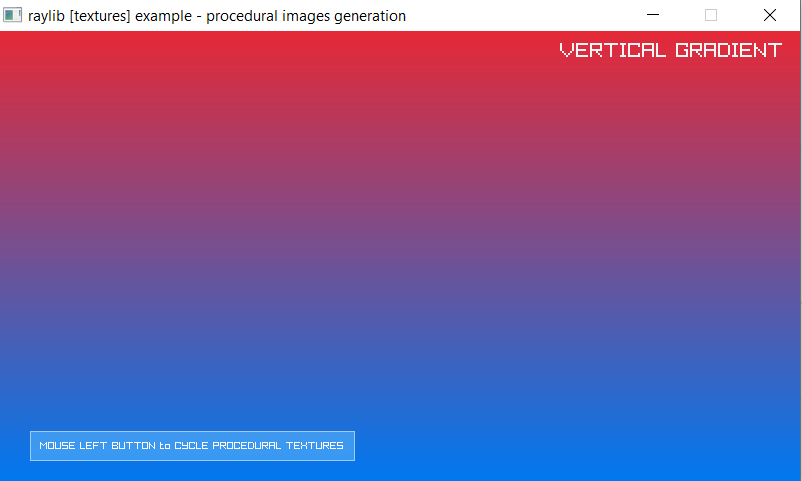
Texture Source
load "raylib.ring"
screenWidth = 800
screenHeight = 600
InitWindow(screenWidth, screenHeight, "raylib [textures] examples - texture source and destination rectangles")
// NOTE: Textures MUST be loaded after Window initialization (OpenGL context is required)
scarfy = LoadTexture("RingLogo.png") // Texture loading
frameWidth = scarfy.width
frameHeight = scarfy.height
// Source rectangle (part of the texture to use for drawing)
sourceRec = Rectangle( 0.0, 0.0, frameWidth, frameHeight )
// Destination rectangle (screen rectangle where drawing part of texture)
destRec = Rectangle( screenWidth/2, screenHeight/2, frameWidth*2, frameHeight*2 )
// Origin of the texture (rotation/scale point), it's relative to destination rectangle size
origin = Vector2( frameWidth, frameHeight )
rotation = 0
SetTargetFPS(60)
while !WindowShouldClose()
rotation = rotation+1
BeginDrawing()
ClearBackground(RAYWHITE)
// NOTE: Using DrawTexturePro() we can easily rotate and scale the part of the texture we draw
// sourceRec defines the part of the texture we use for drawing
// destRec defines the rectangle where our texture part will fit (scaling it to fit)
// origin defines the point of the texture used as reference for rotation and scaling
// rotation defines the texture rotation (using origin as rotation point)
DrawTexturePro(scarfy, sourceRec, destRec, origin, rotation, WHITE)
DrawLine(destRec.x, 0, destRec.x, screenHeight, GRAY)
DrawLine(0, destRec.y, screenWidth, destRec.y, GRAY)
DrawText("(c) Scarfy sprite by Eiden Marsal", screenWidth - 200, screenHeight - 20, 10, GRAY)
EndDrawing()
end
UnloadTexture(scarfy) // Texture unloading
CloseWindow()
Screen Shot:
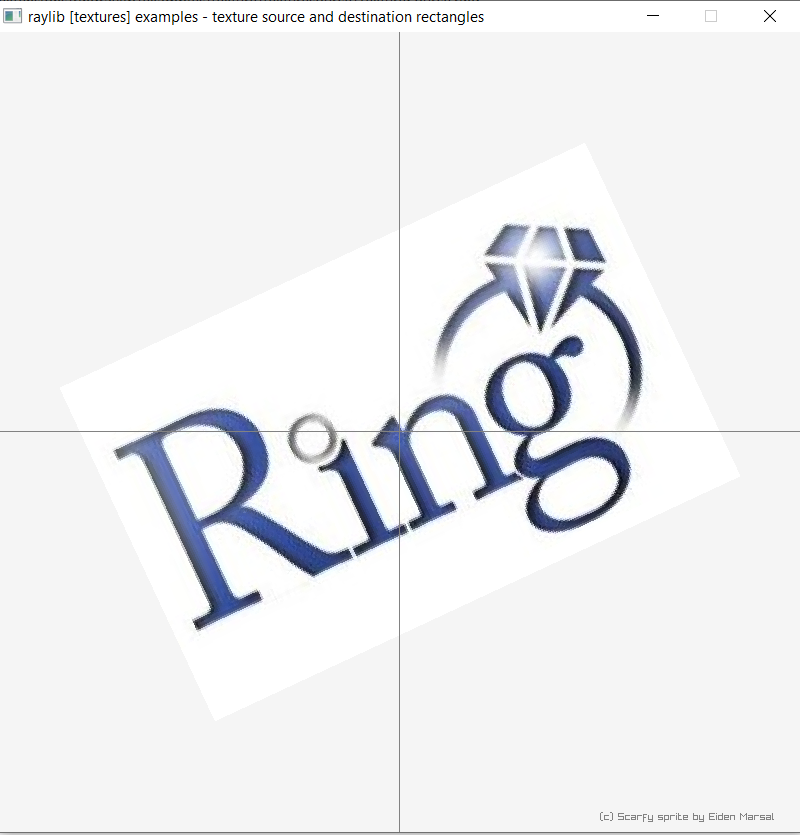
Geometric Shapes
load "raylib.ring"
FOVY_PERSPECTIVE = 45.0
WIDTH_ORTHOGRAPHIC = 10.0
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [models] example - geometric shapes")
camera = Camera3D( 0.0, 10.0, 10.0,
0.0, 0.0, 0.0,
0.0, 1.0, 0.0,
FOVY_PERSPECTIVE, CAMERA_PERSPECTIVE
)
SetTargetFPS(60)
while !WindowShouldClose()
if IsKeyPressed(KEY_SPACE)
if camera.projection = CAMERA_PERSPECTIVE
camera.fovy = WIDTH_ORTHOGRAPHIC
camera.projection = CAMERA_ORTHOGRAPHIC
else
camera.fovy = FOVY_PERSPECTIVE
camera.projection = CAMERA_PERSPECTIVE
ok
ok
BeginDrawing()
ClearBackground(RAYWHITE)
BeginMode3D(camera)
DrawCube(Vector3(-4.0, 0.0, 2.0), 2.0, 5.0, 2.0, RED)
DrawCubeWires(Vector3(-4.0, 0.0, 2.0), 2.0, 5.0, 2.0, GOLD)
DrawCubeWires(Vector3(-4.0, 0.0, -2.0), 3.0, 6.0, 2.0, MAROON)
DrawSphere(Vector3(-1.0, 0.0, -2.0), 1.0, GREEN)
DrawSphereWires(Vector3( 1.0, 0.0, 2.0), 2.0, 16, 16, LIME)
DrawCylinder(Vector3(4.0, 0.0, -2.0), 1.0, 2.0, 3.0, 4, SKYBLUE)
DrawCylinderWires(Vector3(4.0, 0.0, -2.0), 1.0, 2.0, 3.0, 4, DARKBLUE)
DrawCylinderWires(Vector3(4.5, -1.0, 2.0), 1.0, 1.0, 2.0, 6, BROWN)
DrawCylinder(Vector3(1.0, 0.0, -4.0), 0.0, 1.5, 3.0, 8, GOLD)
DrawCylinderWires(Vector3(1.0, 0.0, -4.0), 0.0, 1.5, 3.0, 8, PINK)
DrawGrid(10, 1.0)
EndMode3D()
DrawText("Press Spacebar to switch camera type", 10, GetScreenHeight() - 30, 20, DARKGRAY)
if camera.projection = CAMERA_ORTHOGRAPHIC
DrawText("ORTHOGRAPHIC", 10, 40, 20, BLACK)
else
if camera.projection = CAMERA_PERSPECTIVE
DrawText("PERSPECTIVE", 10, 40, 20, BLACK)
ok
ok
DrawFPS(10, 10)
EndDrawing()
end
CloseWindow()
Screen Shot:
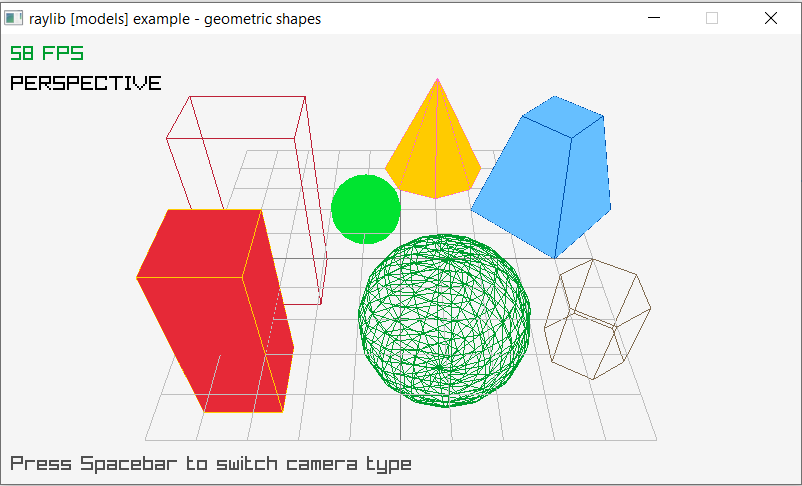
Cubic Map
load "raylib.ring"
screenWidth = 800
screenHeight = 450
InitWindow(screenWidth, screenHeight, "raylib [models] example - cubesmap loading and drawing")
camera = Camera3D( 16.0, 14.0, 16.0,
0.0, 0.0, 0.0,
0.0, 1.0, 0.0,
45.0, CAMERA_PERSPECTIVE )
image = LoadImage("cubicmap.png")
cubicmap = LoadTextureFromImage(image)
mesh = GenMeshCubicmap(image, Vector3( 1.0, 1.0, 1.0 ))
model = LoadModelFromMesh(mesh)
texture = LoadTexture("cubicmap_atlas.png")
setmodelmaterialtexture(model,0,MAP_DIFFUSE,texture)
mapPosition = Vector3( -16.0, 0.0, -8.0 )
UnloadImage(image)
SetTargetFPS(60)
while !WindowShouldClose()
UpdateCamera(camera,CAMERA_ORBITAL)
BeginDrawing()
ClearBackground(RAYWHITE)
BeginMode3D(camera)
DrawModel(model, mapPosition, 1.0, WHITE)
EndMode3D()
DrawTextureEx(cubicmap, Vector2( screenWidth - cubicmap.width*4 - 20, 20 ),
0.0, 4.0, WHITE)
DrawRectangleLines(screenWidth - cubicmap.width*4 - 20, 20, cubicmap.width*4,
cubicmap.height*4, GREEN)
DrawText("cubicmap image used to", 658, 90, 10, GRAY)
DrawText("generate map 3d model", 658, 104, 10, GRAY)
DrawFPS(10, 10)
EndDrawing()
end
UnloadTexture(cubicmap)
UnloadTexture(texture)
UnloadModel(model)
CloseWindow()
Screen Shot:
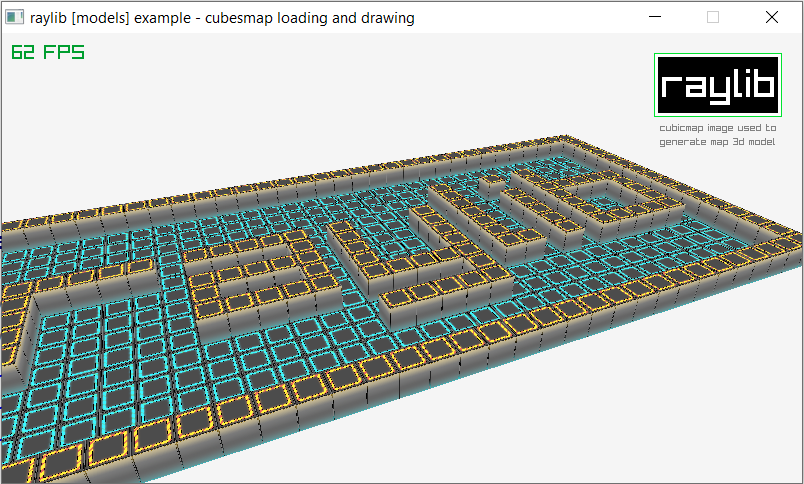
Implementation
The extension exist in the ring/extension/ringraylib5 folder
The supported functions are defined in the ring/extensions/ringraylib5/src/raylib.cf file
The samples exist in the ring/samples/UsingRayLib folder