Building RingQt Applications for WebAssembly¶
In this chapter we will learn about Building RingQt Applications for WebAssembly.
Download Requirements¶
Check the next link : https://doc.qt.io/qt-5/wasm.html
Tested using
Qt (5.15.0) : https://www.qt.io/blog/qt-5.15-released
Emscripten (1.39.7) : https://emscripten.org/docs/getting_started/index.html
emsdk install sdk-fastcomp-1.39.7-64bit
emsdk activate sdk-fastcomp-1.39.7-64bit
Check Emscripten installation
em++ --version
Output
emcc (Emscripten gcc/clang-like replacement) 1.39.7
(commit 24d88487f47629fac9d4acd231497a3a412bdee8)
Copyright (C) 2014 the Emscripten authors (see AUTHORS.txt)
This is free and open source software under the MIT license.
There is NO warranty; not even for MERCHANTABILITY or FITNESS FOR A
PARTICULAR PURPOSE.
Using Ring2EXE¶
We can use Ring2EXE to quickly prepare Qt project for our application
Example:
ring2exe myapp.ring -dist -webassemblyqt
Note
We can use the Distribute Menu in Ring Notepad
Tip
The option ( Prepare Qt project for WebAssembly ) in the Distribute Menu
The Qt project for your Ring application¶
After using Ring2EXE or the Distribute Menu in Ring Notepad
Using the Qt Creator Open the generated Qt project
Folder : target/webassembly/qtproject
Project file : project.pro
Using Qt Creator, You will find the compiled Ring application in the resources (YourAppName.ringo)
This file (Ring Object File) is generated by the Ring compiler using
ring YourAppName.ring -go -norun
You can build your application using Qt Creator
You can add your application images to the resources
Or You can use any text editor (Notepad) and modify : project.qrc
To find images from your Ring application, You need to use the file name in resources
Example
if isWebAssembly()
mypic = new QPixmap(":/cards.jpg")
else
mypic = new QPixmap("cards.jpg")
ok
Comments about developing for WebAssembly using RingQt¶
The main project file is main.cpp
This file load Ring Compiler/Virtual Machine and RingQt
Then get the Ring Object File during the runtime from the resources
Then run the Ring Object File (ringapp.ringo) using the Ring VM
Through main.cpp you can extract more files from the resources to temp. folder once you add them (create projects with many files).
use if isWebAssembly() when you want to modify the code just for WebAssembly
Example:
if isWebAssembly()
// WebAssembly code
else
// other platforms
ok
When you deal with Qt Classes you can determine the images from resources (you don’t need to copy them using main.cpp)
Example:
if isWebAssembly()
mypic = new QPixmap(":/cards.jpg")
else
mypic = new QPixmap("cards.jpg")
ok
Now RingQt comes with the AppFile() function to determine the file name
Example:
mypic = new QPixmap(AppFile("cards.jpg")) # Desktop, Android or WebAssembly
When you update your project code, You don’t have to use Ring2EXE to generate the Qt project again
Just use the Distribute Menu in Ring Notepad and select (Generate Ring Object File)
Then copy the YourAppName.ringo file to target/webassembly/qtproject folder and accept replacing files.
If your application folder contains a Qt resource file (project.qrc)
Then when you use Ring2EXE or Ring Notepad (Distribute - Prepare Qt project for WebAssembly) the resource file will be used
See ring/applications/cards game as an example.
Use stdlibcore.ring instead of stdlib.ring when using StdLib functions
Use ClocksPerSecond() function instead of typing the value (1000)
Nested events loops are not supported, use events for dialogs instead of calling the exec() method
Using Sleep() or ProcessEvents() doesn’t provide the expected results, use Qt Timers.
We don’t have a direct access to the File System because the applications are executed in a secure environment
Tip
We can use special functions for Uploading/Downloading files (See FileContent sample)
Dialogs¶
See the folder: ring/samples/UsingQtWASM
Folders:
ColorDialog
FontDialog
FileDialog
FileContent
Online Applications¶
Hello World : https://ring-lang.github.io/web/helloworld/project.html
Matching Game : https://ring-lang.github.io/web/matching/project.html
Pairs Game : https://ring-lang.github.io/web/pairs/project.html
Othello Game : https://ring-lang.github.io/web/othello/project.html
Game of Life : https://ring-lang.github.io/web/gameoflife/project.html
Form Designer : https://ring-lang.github.io/web/formdesigner/project.html
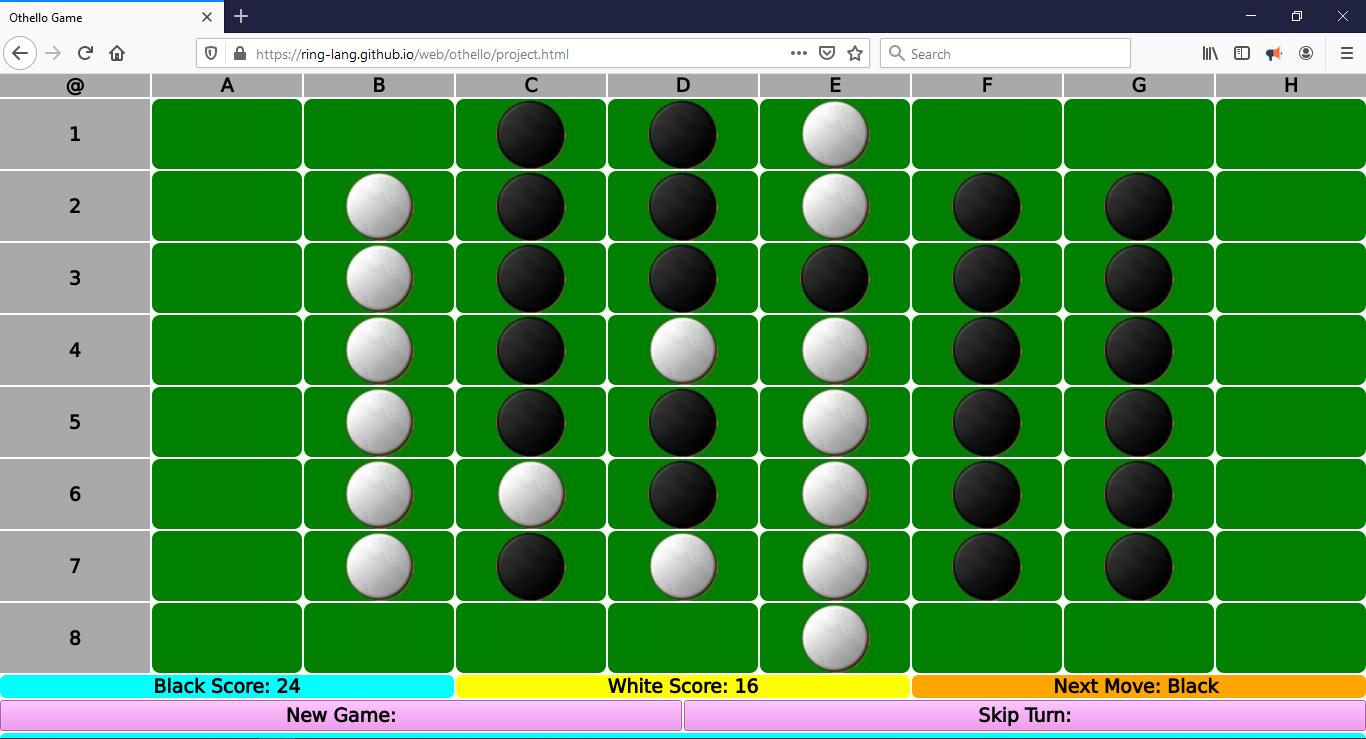