What is new in Ring 1.3?¶
In this chapter we will learn about the changes and new features in Ring 1.3 release.
List of changes and new features¶
Ring 1.3 comes with many new features
Better RingQt
Better Ring Notepad
Ring mode for Emacs Editor
Better StdLib
Better Loop/Exit Command
New Functions
Return Self by Reference
Using ‘<’ and ‘:’ operators as ‘from’ keyword
Embedding Ring in Ring without sharing the State
RingZip Library
Form Designer
Better RingQt¶
(1) Another version of QPixMap class is added (QPixMap2) which takes (int width,int height) during object init.
Example:
Load "guilib.ring"
New qapp
{
win1 = new qwidget()
{
setwindowtitle("Drawing using QPixMap")
setgeometry(100,100,500,500)
label1 = new qlabel(win1)
{
setgeometry(10,10,400,400)
settext("")
}
imageStock = new qlabel(win1)
{
image = new qPixMap2(200,200)
color = new qcolor() {
setrgb(255,255,255,255)
}
pen = new qpen() {
setcolor(color)
setwidth(10)
}
new qpainter() {
begin(image)
setpen(pen)
drawline(0,0,200,200)
drawline(200,0,0,200)
endpaint()
}
setpixmap(image)
}
show()
}
exec()
}
Screen Shot:
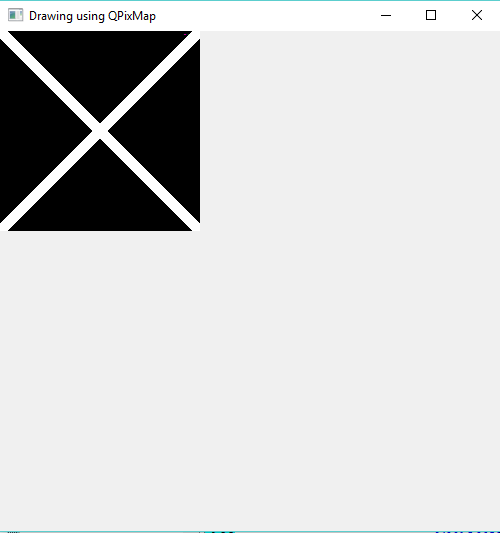
The Objects Library is updated to include the next functions
Last_WindowID()
Open_WindowNoShow()
Open_WindowAndLink()
Also the class name (WindowViewBase) is changed to (WindowsViewParent).
In The next code for example the Open_WindowAndLink() will create an object from the SecondWindowController Class Then will add the Method SecondWindow() to the FirstWindowController Class Also will add the Method FirstWindow() to the SecondWindowController Class
So the SendMessage() method in FirstWindowController class can use the SecondWindow() method to access the object.
class firstwindowController from windowsControllerParent
oView = new firstwindowView
func OpenSecondWindow
Open_WindowAndLink(:SecondWindowController,self)
func SendMessage
if IsSecondWindow()
SecondWindow().setMessage("Message from the first window")
ok
func setMessage cMessage
oView.Label1.setText(cMessage)
The next classes are added to RingQt
QPixMap2
QScrollArea
QSplitter
QCompleter
QCompleter2
QCompleter3
QProcess
QMdiArea
QMdiSubWindow
QCursor
QListView
QDesktopServices
Many constants are defined in qt.rh (loaded by guilib.ring)
New Classes names - Index Start from 1
We added new classes to RingQt - another version of classes where the class names doesn’t start with the “q” letter Also updated methods so the index start from 1 when we deal with the GUI controls like
ComboBox
ListWidget
TableWidget
TreeWidget
These classes are inside guilib.ring under the package name : System.GUI
To use it
load "guilib.ring"
import System.GUI
This doesn’t have any effect on our previous code, It’s just another choice for better code that is consistent with Ring rules.
Also the form designer is updated to provide us the choice between using classes where (index start from 0) or (index start from 1)
Example (Uses the Form Designer)
Better Ring Notepad¶
Using QPlainTextEdit instead of QTextEdit
Displaying the line number for each line in the source code file.
Screen Shot:
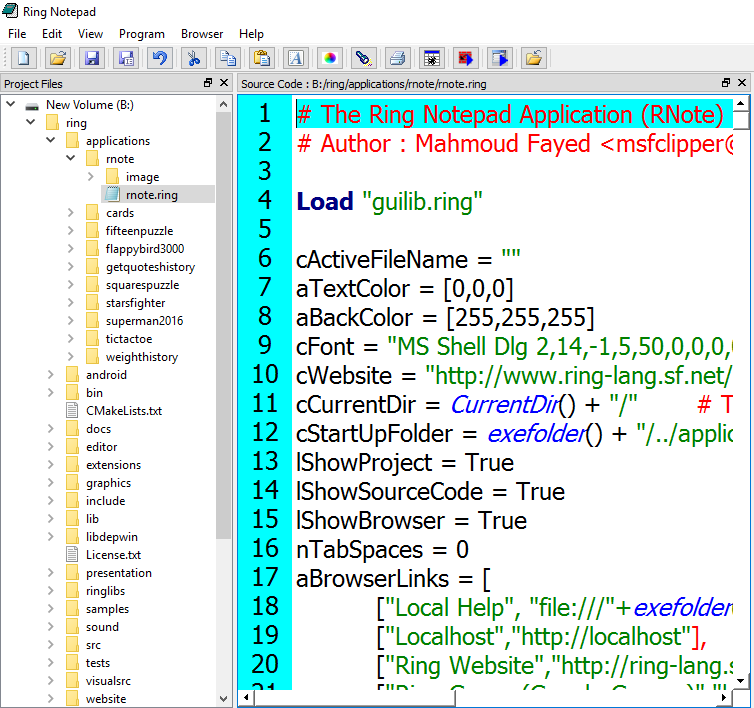
Auto-Complete for Ring functions names, classes and words in the opened file.
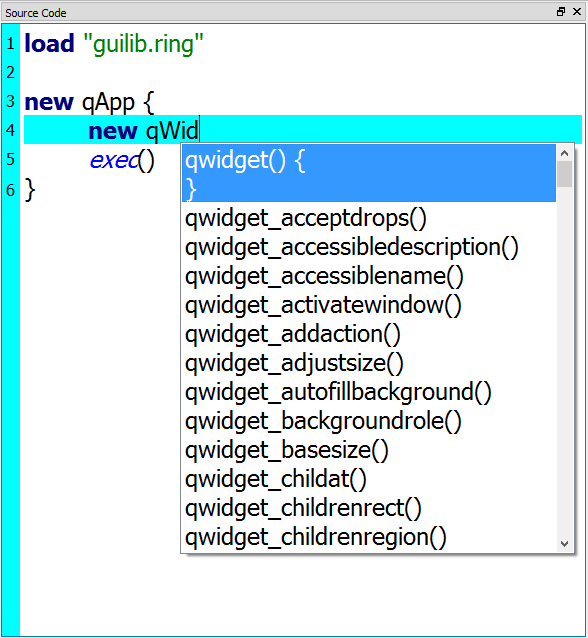
Functions and Methods List
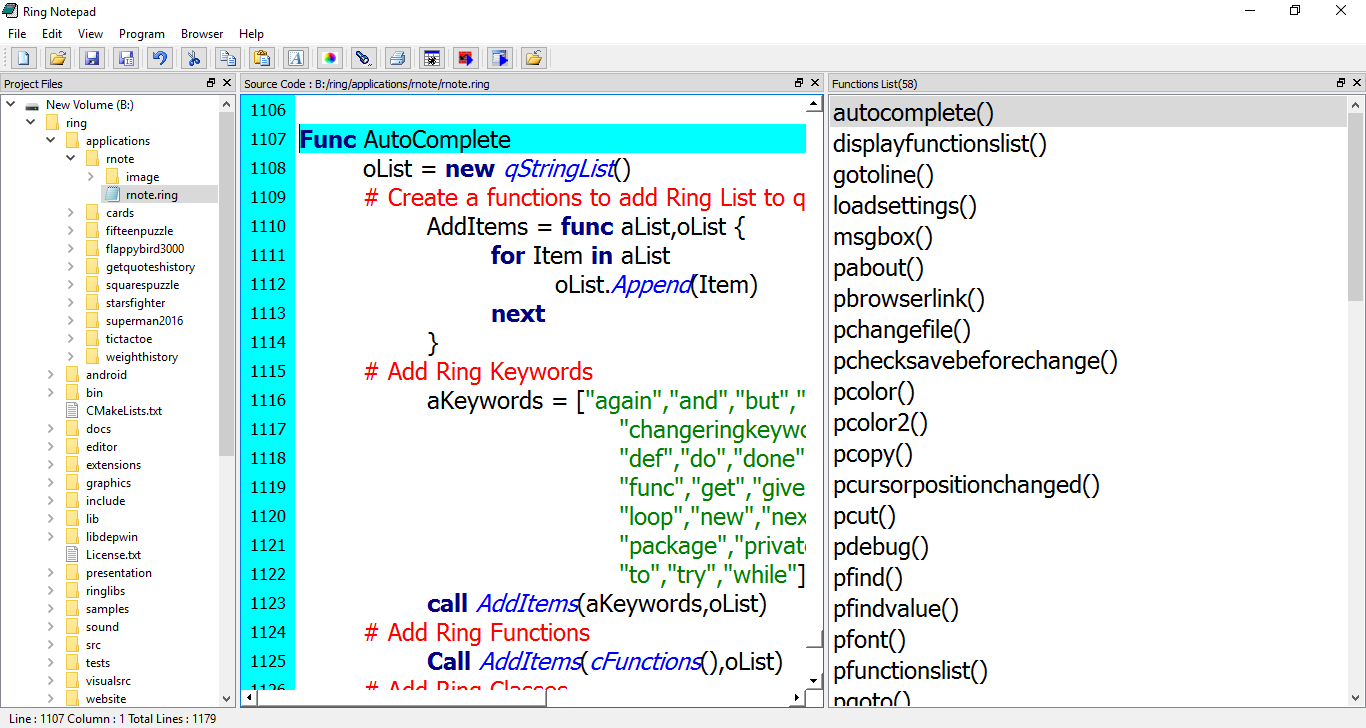
Output Window
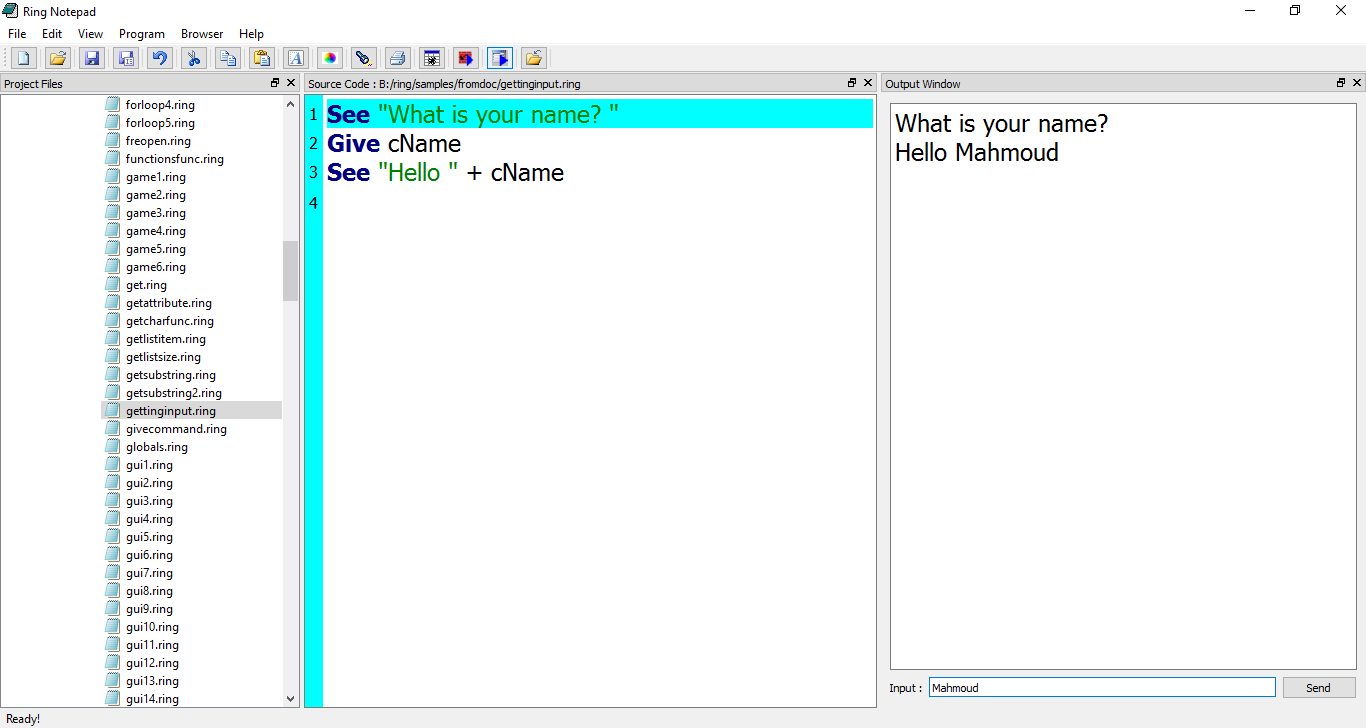
Classes List
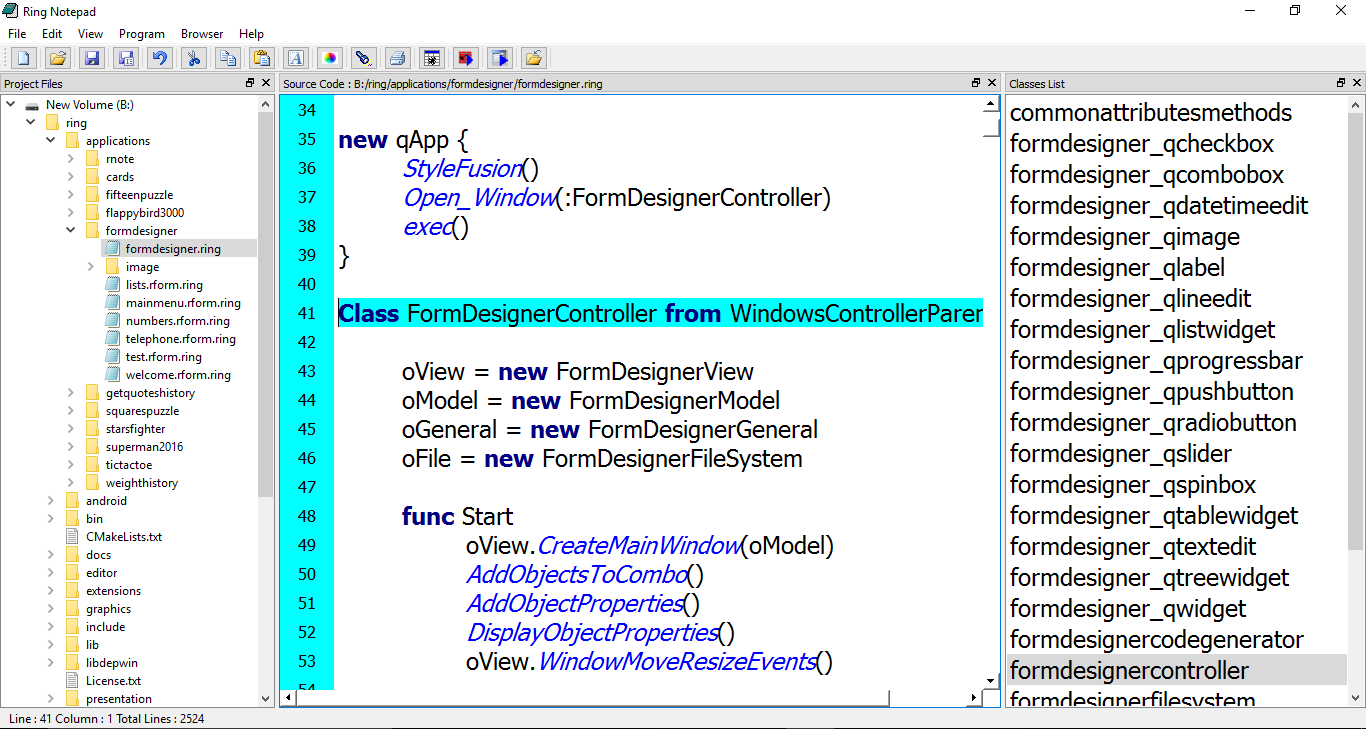
Change the Current Style
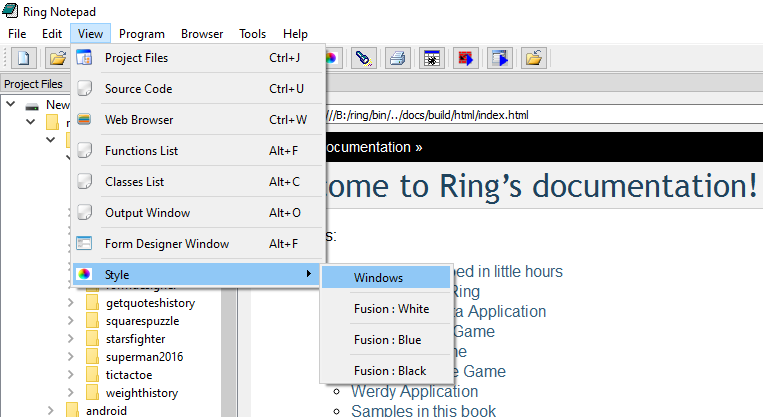
Better StdLib¶
The StdLib is updated to include the next functions
SplitMany()
JustFilePath()
JustFileName()
Better Loop|Exit Command¶
The Loop|Exit command is updated to accept Expressions after the command (not only numbers).
The syntax:
Loop|Exit [Number]
Changed to
Loop|Exit [Expression]
Example
XLoop = 2 # The outer loop
YLoop = 1 # The first inner loop
for x = 1 to 10
for y = 1 to 10
see "x=" + x + " y=" + y + nl
if x = 3 and y = 5
exit XLoop
ok
next
next
New Functions¶
PackageName() function
Swap() function
Example:
aList = [:one,:two,:four,:three]
see aList
see copy("*",50) + nl
swap(aList,3,4)
see aList
Output
one
two
four
three
**************************************************
one
two
three
four
Return Self by Reference¶
In this release, using Return Self in class methods will return the object by reference.
Example:
mylist = [new mytest() {
see self
x = 20
see self
}]
see mylist
class mytest
x = 15
func init
return self # Return by reference
Output
x: 15.000000
x: 20.000000
x: 20.000000
Using ‘<’ and ‘:’ operators as ‘from’ keyword¶
In this release of the Ring language we can use the ‘<’ and ‘:’ operators as the ‘from’ keyword
Syntax (1):
class Cat from Animal
Syntax (2):
class Cat < Animal
Syntax (3):
class Cat : Animal
Embedding Ring in Ring without sharing the State¶
From Ring 1.0 we already have functions for embedding Ring in the C language. Also we can execute Ring code inside Ring programs using the eval() function. In this release we provide functions for embedding Ring in Ring programs without sharing the state.
Advantages:
Quick integration for Ring programs and applications together without conflicts.
Execute and run Ring code in safe environments that we can trace.
Example:
pState = ring_state_init()
ring_state_runcode(pState,"See 'Hello, World!'+nl")
ring_state_runcode(pState,"x = 10")
pState2 = ring_state_init()
ring_state_runcode(pState2,"See 'Hello, World!'+nl")
ring_state_runcode(pState2,"x = 20")
ring_state_runcode(pState,"see x +nl")
ring_state_runcode(pState2,"see x +nl")
v1 = ring_state_findvar(pState,"x")
v2 = ring_state_findvar(pState2,"x")
see v1[3] + nl
see V2[3] + nl
ring_state_delete(pState)
ring_state_delete(pState2)
Output:
Hello, World!
Hello, World!
10
20
10
20
RingZip Library¶
Ring 1.3 comes with the RingZip library for creating, modifying and extracting *.zip files.
Example (1): Create myfile.zip contains 4 files
load "ziplib.ring"
oZip = zip_openfile("myfile.zip",'w')
zip_addfile(oZip,"test.c")
zip_addfile(oZip,"zip.c")
zip_addfile(oZip,"zip.h")
zip_addfile(oZip,"miniz.h")
zip_close(oZip)
Example (2): Extract myfile.zip to myfolder folder.
load "ziplib.ring"
zip_extract_allfiles("myfile.zip","myfolder")
Example (3): Print file names in the myfile.zip
load "ziplib.ring"
oZip = zip_openfile("myfile.zip",'r')
for x=1 to zip_filescount(oZip)
see zip_getfilenamebyindex(oZip,x) + nl
next
zip_close(oZip)
Example (4) : Using Classes instead of Functions
load "ziplib.ring"
new Zip {
SetFileName("myfile.zip")
Open("w")
AddFile("test.c")
AddFile("zip.c")
AddFile("zip.h")
AddFile("miniz.h")
Close()
}
Form Designer¶
Ring 1.3 comes with the Form Designer to quickly design your GUI application windows/forms and generate the Ring source code.
It’s written in Ring (Around 8000 Lines of code) using Object-Oriented Programming and Meta-Programming.
We can run the From Designer from Ring Notepad
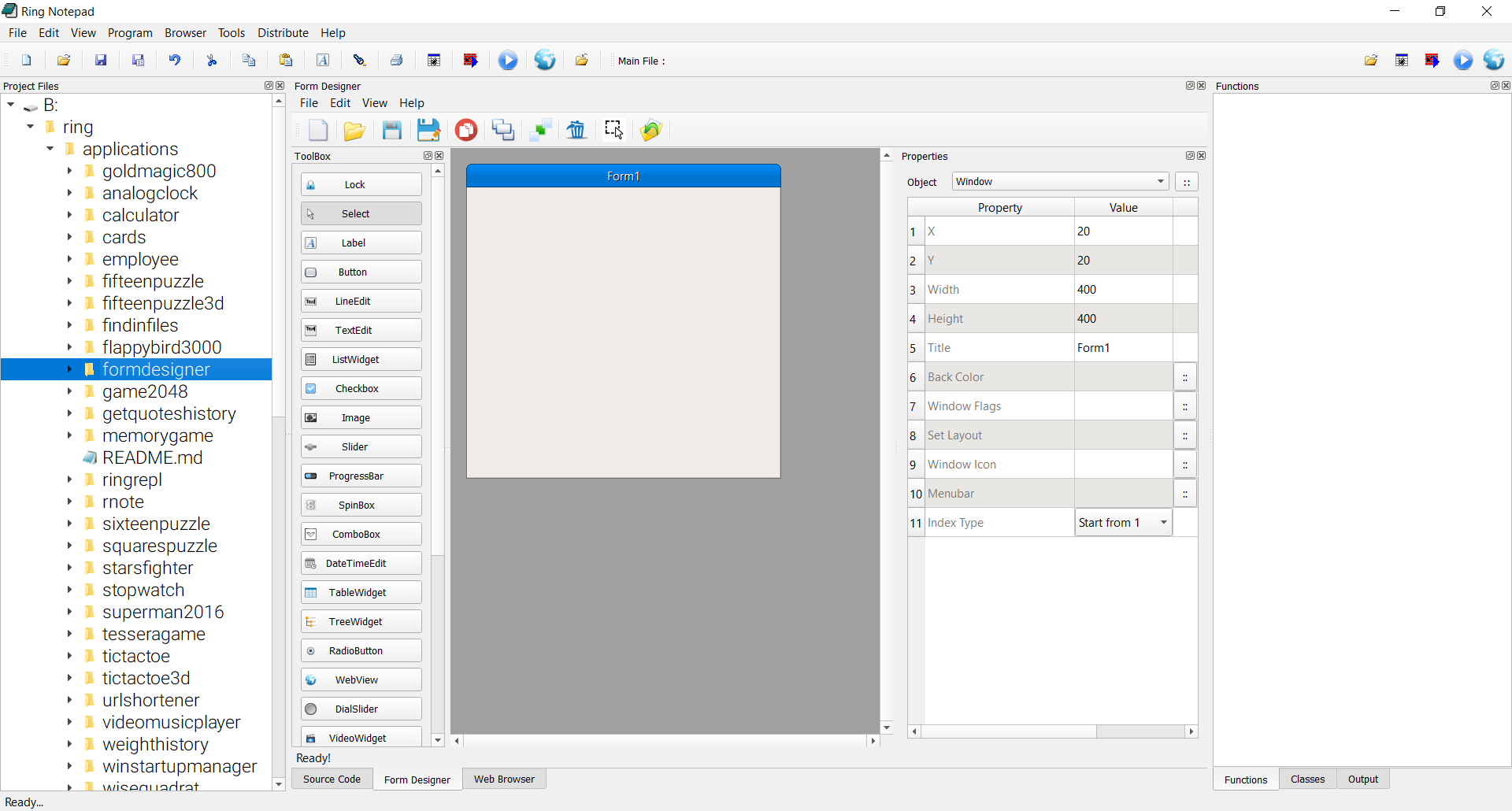
Also we can run the Form Designer in another window.
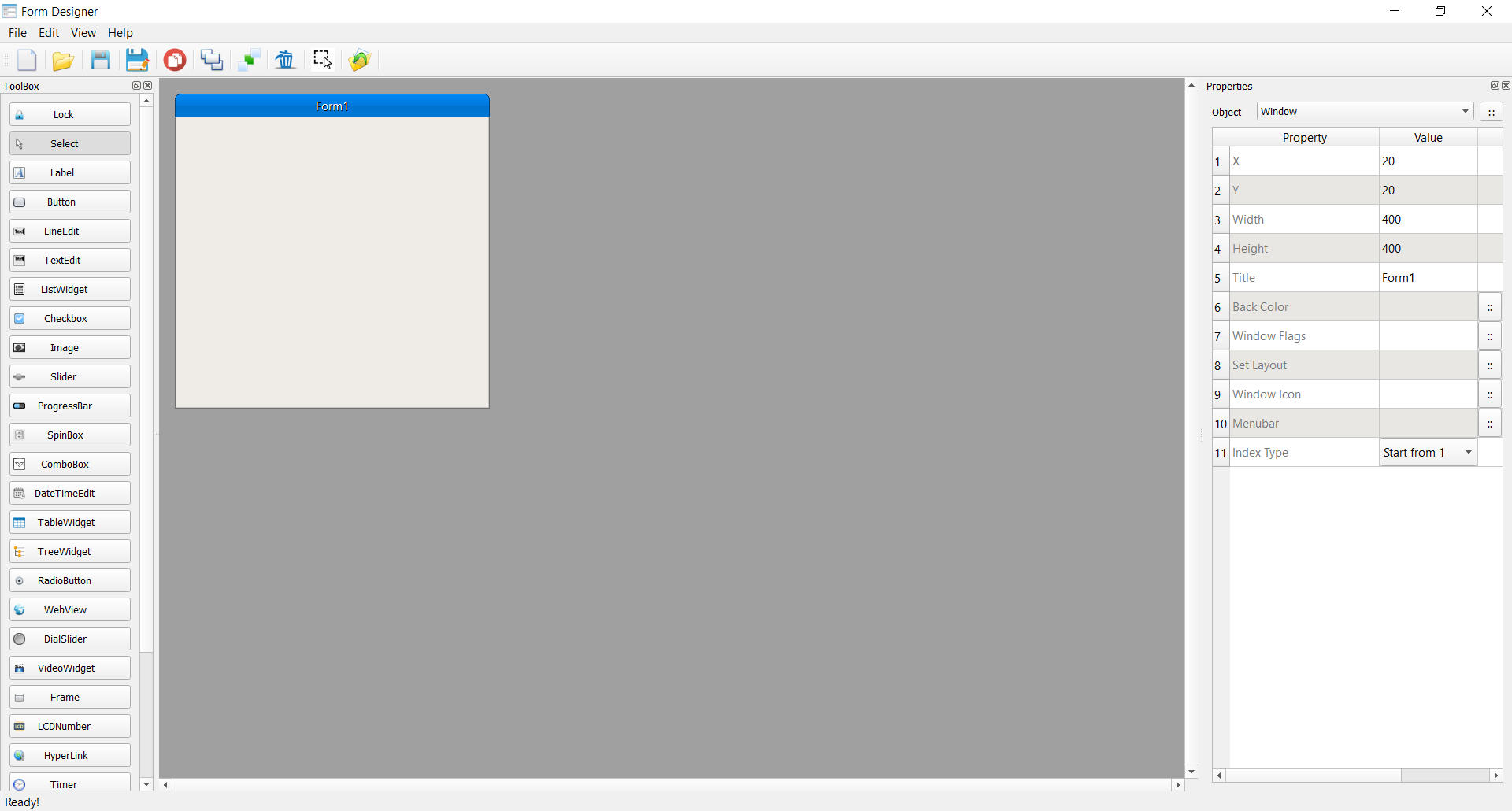